mirror of
https://github.com/trezor/trezor-firmware.git
synced 2025-07-02 12:52:34 +00:00
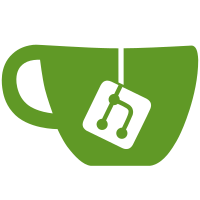
Holding confirm button at home screen asks user whether they wish to lock the TREZOR (clear the cached PIN and passphrase and show the screensaver). This is identical behaviour to the ClearSession message.
110 lines
2.5 KiB
C
110 lines
2.5 KiB
C
/*
|
|
* This file is part of the TREZOR project.
|
|
*
|
|
* Copyright (C) 2014 Pavol Rusnak <stick@satoshilabs.com>
|
|
*
|
|
* This library is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU Lesser General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public License
|
|
* along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include "trezor.h"
|
|
#include "oled.h"
|
|
#include "bitmaps.h"
|
|
#include "util.h"
|
|
#include "usb.h"
|
|
#include "setup.h"
|
|
#include "storage.h"
|
|
#include "layout.h"
|
|
#include "layout2.h"
|
|
#include "rng.h"
|
|
#include "buttons.h"
|
|
|
|
uint32_t __stack_chk_guard;
|
|
|
|
void __attribute__((noreturn)) __stack_chk_fail(void)
|
|
{
|
|
layoutDialog(&bmp_icon_error, NULL, NULL, NULL, "Stack smashing", "detected.", NULL, "Please unplug", "the device.", NULL);
|
|
for (;;) {} // loop forever
|
|
}
|
|
|
|
void check_lock_screen(void)
|
|
{
|
|
buttonUpdate();
|
|
|
|
// wake from screensaver on any button
|
|
if (layoutLast == layoutScreensaver && (button.NoUp || button.YesUp)) {
|
|
layoutHome();
|
|
return;
|
|
}
|
|
|
|
// button held for long enough
|
|
if (layoutLast == layoutHome && button.NoDown >= 500000) {
|
|
|
|
layoutDialog(&bmp_icon_question, "Cancel", "Lock Device", NULL, "Do you really want to", "lock your TREZOR?", NULL, NULL, NULL, NULL);
|
|
|
|
// wait until NoButton is released
|
|
usbTiny(1);
|
|
do {
|
|
usbDelay(3300);
|
|
buttonUpdate();
|
|
} while (!button.NoUp);
|
|
|
|
// wait for confirmation/cancellation of the dialog
|
|
do {
|
|
usbDelay(3300);
|
|
buttonUpdate();
|
|
} while (!button.YesUp && !button.NoUp);
|
|
usbTiny(0);
|
|
|
|
if (button.YesUp) {
|
|
// lock the screen
|
|
session_clear(true);
|
|
layoutScreensaver();
|
|
} else {
|
|
// resume homescreen
|
|
layoutHome();
|
|
}
|
|
}
|
|
}
|
|
|
|
int main(void)
|
|
{
|
|
__stack_chk_guard = random32();
|
|
#ifndef APPVER
|
|
setup();
|
|
oledInit();
|
|
#else
|
|
setupApp();
|
|
#endif
|
|
#if DEBUG_LINK
|
|
oledSetDebug(1);
|
|
storage_reset(); // wipe storage if debug link
|
|
storage_reset_uuid();
|
|
storage_commit();
|
|
storage_clearPinArea(); // reset PIN failures if debug link
|
|
#endif
|
|
|
|
oledDrawBitmap(40, 0, &bmp_logo64);
|
|
oledRefresh();
|
|
|
|
storage_init();
|
|
layoutHome();
|
|
usbInit();
|
|
for (;;) {
|
|
usbPoll();
|
|
check_lock_screen();
|
|
}
|
|
|
|
return 0;
|
|
}
|