mirror of
https://github.com/trezor/trezor-firmware.git
synced 2024-10-10 18:09:00 +00:00
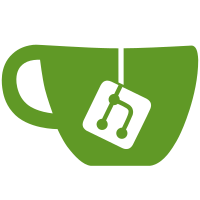
Every 4294967295 milliseconds (2 ^ 32 - 1), system_millis will overflow. This means that every 49.71 days, system_millis will reset to zero. Comparisons like `system_millis < (system_millis + 1)` would fail if the latter had overflown and the former had not. This is non-critical because the worst case is that one second could be skipped or the screen could lock early. This poses no threat to the exponential backoff used for protection against brute force.
65 lines
1.5 KiB
C
65 lines
1.5 KiB
C
/*
|
|
* This file is part of the TREZOR project.
|
|
*
|
|
* Copyright (C) 2016 Saleem Rashid <trezor@saleemrashid.com>
|
|
*
|
|
* This library is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU Lesser General Public License as published by
|
|
* the Free Software Foundation, either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public License
|
|
* along with this library. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
|
|
#include "timer.h"
|
|
|
|
#include <libopencm3/stm32/rcc.h>
|
|
#include <libopencm3/cm3/systick.h>
|
|
|
|
/* 1 tick = 1 ms */
|
|
volatile uint32_t system_millis;
|
|
|
|
/* Screen timeout */
|
|
uint32_t system_millis_lock_start;
|
|
|
|
/*
|
|
* Initialise the Cortex-M3 SysTick timer
|
|
*/
|
|
void timer_init(void) {
|
|
system_millis = 0;
|
|
|
|
/*
|
|
* MCU clock (120 MHz) as source
|
|
*
|
|
* (120 MHz / 8) = 15 clock pulses
|
|
*
|
|
*/
|
|
systick_set_clocksource(STK_CSR_CLKSOURCE_AHB_DIV8);
|
|
STK_CVR = 0;
|
|
|
|
/*
|
|
* 1 tick = 1 ms @ 120 MHz
|
|
*
|
|
* (15 clock pulses * 1000 ms) = 15000 clock pulses
|
|
*
|
|
* Send an interrupt every (N - 1) clock pulses
|
|
*/
|
|
systick_set_reload(14999);
|
|
|
|
/* SysTick as interrupt */
|
|
systick_interrupt_enable();
|
|
|
|
systick_counter_enable();
|
|
}
|
|
|
|
void sys_tick_handler(void) {
|
|
system_millis++;
|
|
}
|