mirror of
https://github.com/trezor/trezor-firmware.git
synced 2024-10-12 10:58:59 +00:00
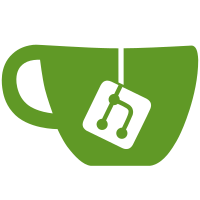
all debug input signals are now channels, and DebugLinkDecision handler waits until the input was consumed. This means that the input events are queued; originally, if an input event arrived before the previous was consumed, the previous input would be lost. reset words and their positions are now also channels, and DebugLinkGetState can wait for their updates, if required
108 lines
3.2 KiB
Protocol Buffer
108 lines
3.2 KiB
Protocol Buffer
syntax = "proto2";
|
|
package hw.trezor.messages.debug;
|
|
|
|
// Sugar for easier handling in Java
|
|
option java_package = "com.satoshilabs.trezor.lib.protobuf";
|
|
option java_outer_classname = "TrezorMessageDebug";
|
|
|
|
import "messages-common.proto";
|
|
|
|
/**
|
|
* Request: "Press" the button on the device
|
|
* @start
|
|
* @next Success
|
|
*/
|
|
message DebugLinkDecision {
|
|
optional bool yes_no = 1; // true for "Confirm", false for "Cancel"
|
|
optional bool up_down = 2; // true for scroll up, false for scroll down
|
|
optional string input = 3; // keyboard input
|
|
}
|
|
|
|
/**
|
|
* Request: Computer asks for device state
|
|
* @start
|
|
* @next DebugLinkState
|
|
*/
|
|
message DebugLinkGetState {
|
|
optional bool wait_word_list = 1; // Trezor T only - wait until mnemonic words are shown
|
|
optional bool wait_word_pos = 2; // Trezor T only - wait until reset word position is requested
|
|
}
|
|
|
|
/**
|
|
* Response: Device current state
|
|
* @end
|
|
*/
|
|
message DebugLinkState {
|
|
optional bytes layout = 1; // raw buffer of display
|
|
optional string pin = 2; // current PIN, blank if PIN is not set/enabled
|
|
optional string matrix = 3; // current PIN matrix
|
|
optional bytes mnemonic_secret = 4; // current mnemonic secret
|
|
optional hw.trezor.messages.common.HDNodeType node = 5; // current BIP-32 node
|
|
optional bool passphrase_protection = 6; // is node/mnemonic encrypted using passphrase?
|
|
optional string reset_word = 7; // word on device display during ResetDevice workflow
|
|
optional bytes reset_entropy = 8; // current entropy during ResetDevice workflow
|
|
optional string recovery_fake_word = 9; // (fake) word on display during RecoveryDevice workflow
|
|
optional uint32 recovery_word_pos = 10; // index of mnemonic word the device is expecting during RecoveryDevice workflow
|
|
optional uint32 reset_word_pos = 11; // index of mnemonic word the device is expecting during ResetDevice workflow
|
|
optional uint32 mnemonic_type = 12; // current mnemonic type (BIP-39/SLIP-39)
|
|
}
|
|
|
|
/**
|
|
* Request: Ask device to restart
|
|
* @start
|
|
*/
|
|
message DebugLinkStop {
|
|
}
|
|
|
|
/**
|
|
* Response: Device wants host to log event
|
|
* @ignore
|
|
*/
|
|
message DebugLinkLog {
|
|
optional uint32 level = 1;
|
|
optional string bucket = 2;
|
|
optional string text = 3;
|
|
}
|
|
|
|
/**
|
|
* Request: Read memory from device
|
|
* @start
|
|
* @next DebugLinkMemory
|
|
*/
|
|
message DebugLinkMemoryRead {
|
|
optional uint32 address = 1;
|
|
optional uint32 length = 2;
|
|
}
|
|
|
|
/**
|
|
* Response: Device sends memory back
|
|
* @end
|
|
*/
|
|
message DebugLinkMemory {
|
|
optional bytes memory = 1;
|
|
}
|
|
|
|
/**
|
|
* Request: Write memory to device.
|
|
* WARNING: Writing to the wrong location can irreparably break the device.
|
|
* @start
|
|
* @next Success
|
|
* @next Failure
|
|
*/
|
|
message DebugLinkMemoryWrite {
|
|
optional uint32 address = 1;
|
|
optional bytes memory = 2;
|
|
optional bool flash = 3;
|
|
}
|
|
|
|
/**
|
|
* Request: Erase block of flash on device
|
|
* WARNING: Writing to the wrong location can irreparably break the device.
|
|
* @start
|
|
* @next Success
|
|
* @next Failure
|
|
*/
|
|
message DebugLinkFlashErase {
|
|
optional uint32 sector = 1;
|
|
}
|