mirror of
https://github.com/trezor/trezor-firmware.git
synced 2025-07-07 07:12:34 +00:00
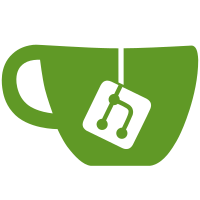
This is to avoid including app-specific functionality in storage and avoid circular imports. The following policy is now in effect: modules from `storage` namespace must not import from `apps` namespace. In most files, the change only involves changing import paths. A minor refactor was needed in case of webauthn: basic get/set/delete functionality was left in storage.webauthn, and more advanced logic on top of it was moved to apps.webauthn.resident_credentials. A significant refactor was needed for sd_salt, where application (and UI) logic was tightly coupled with the IO code. This is now separated, and storage.sd_salt deals exclusively with the IO side, while the app/UI logic is implemented on top of it in apps.common.sd_salt and apps.management.sd_protect.
76 lines
2.4 KiB
Python
76 lines
2.4 KiB
Python
import storage.sd_salt
|
|
from storage.sd_salt import SD_CARD_HOT_SWAPPABLE, SdSaltMismatch
|
|
from trezor import io, ui, wire
|
|
from trezor.ui.text import Text
|
|
|
|
from apps.common.confirm import confirm
|
|
|
|
if False:
|
|
from typing import Optional
|
|
|
|
|
|
class SdProtectCancelled(Exception):
|
|
pass
|
|
|
|
|
|
async def _wrong_card_dialog(ctx: wire.GenericContext) -> bool:
|
|
text = Text("SD card protection", ui.ICON_WRONG)
|
|
text.bold("Wrong SD card.")
|
|
text.br_half()
|
|
if SD_CARD_HOT_SWAPPABLE:
|
|
text.normal("Please insert the", "correct SD card for", "this device.")
|
|
btn_confirm = "Retry" # type: Optional[str]
|
|
btn_cancel = "Abort"
|
|
else:
|
|
text.normal("Please unplug the", "device and insert the", "correct SD card.")
|
|
btn_confirm = None
|
|
btn_cancel = "Close"
|
|
|
|
return await confirm(ctx, text, confirm=btn_confirm, cancel=btn_cancel)
|
|
|
|
|
|
async def _insert_card_dialog(ctx: wire.GenericContext) -> None:
|
|
text = Text("SD card protection", ui.ICON_WRONG)
|
|
text.bold("SD card required.")
|
|
text.br_half()
|
|
if SD_CARD_HOT_SWAPPABLE:
|
|
text.normal("Please insert your", "SD card.")
|
|
btn_confirm = "Retry" # type: Optional[str]
|
|
btn_cancel = "Abort"
|
|
else:
|
|
text.normal("Please unplug the", "device and insert your", "SD card.")
|
|
btn_confirm = None
|
|
btn_cancel = "Close"
|
|
|
|
if not await confirm(ctx, text, confirm=btn_confirm, cancel=btn_cancel):
|
|
raise SdProtectCancelled
|
|
|
|
|
|
async def sd_write_failed_dialog(ctx: wire.GenericContext) -> bool:
|
|
text = Text("SD card protection", ui.ICON_WRONG, ui.RED)
|
|
text.normal("Failed to write data to", "the SD card.")
|
|
return await confirm(ctx, text, confirm="Retry", cancel="Abort")
|
|
|
|
|
|
async def ensure_sd_card(ctx: wire.GenericContext) -> None:
|
|
sd = io.SDCard()
|
|
while not sd.power(True):
|
|
await _insert_card_dialog(ctx)
|
|
|
|
|
|
async def request_sd_salt(
|
|
ctx: wire.GenericContext = wire.DUMMY_CONTEXT
|
|
) -> Optional[bytearray]:
|
|
while True:
|
|
ensure_sd_card(ctx)
|
|
try:
|
|
return storage.sd_salt.load_sd_salt()
|
|
except SdSaltMismatch as e:
|
|
if not await _wrong_card_dialog(ctx):
|
|
raise SdProtectCancelled from e
|
|
except OSError:
|
|
# This happens when there is both old and new salt file, and we can't move
|
|
# new salt over the old salt. If the user clicks Retry, we will try again.
|
|
if not await sd_write_failed_dialog(ctx):
|
|
raise
|