mirror of
https://github.com/trezor/trezor-firmware.git
synced 2024-11-10 01:30:19 +00:00
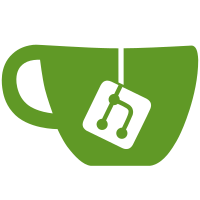
Removed oldest v1 style of firmware signature and presence checks. Added debug helpers for T1 signatures. Support for v2 and v3 signatures, but can only update FW to v3-style signed. Support for debugging T1 signatures. Scripts and README for debugging v2/v3 FW signing scheme. Firmware in GetFeatures counts only v3 signatures as signed. Add documentation and comments about signing schemes like a sane person
23 lines
729 B
Python
Executable File
23 lines
729 B
Python
Executable File
#!/usr/bin/env python3
|
|
import sys
|
|
from hashlib import sha256
|
|
|
|
import ecdsa
|
|
|
|
# arg 1 - hex digest of firmware header with zeroed sigslots
|
|
# arg 2 - public key (compressed or uncompressed)
|
|
# arg 3 - signature 64 bytes in hex
|
|
digest = bytes.fromhex(sys.argv[1])
|
|
assert len(digest) == 32
|
|
public_key = bytes.fromhex(sys.argv[2])
|
|
sig = bytes.fromhex(sys.argv[3])
|
|
assert len(sig) == 64
|
|
# 0x18 - coin info, 0x20 - length of digest following
|
|
prefix = b"\x18Bitcoin Signed Message:\n\x20"
|
|
message_predigest = prefix + digest
|
|
message = sha256(message_predigest).digest()
|
|
|
|
vk = ecdsa.VerifyingKey.from_string(public_key, curve=ecdsa.SECP256k1, hashfunc=sha256)
|
|
result = vk.verify(sig, message)
|
|
print("Signature verification result", result)
|