mirror of
https://github.com/trezor/trezor-firmware.git
synced 2024-11-19 22:18:13 +00:00
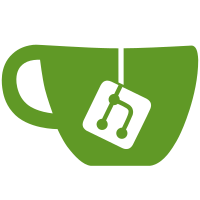
- uses protobuff messages only for the transaction construction data - forward compatible. Not stable on Monero side, serialization format of the cryptonote serialization could be changed in the future (new fields), CN serialization does not support versioning, thus it is not forward compatible. - MoneroTransactionDestinationEntry, MoneroTransactionSourceEntry have to be defined in the root level as they are used in various protocol messages
438 lines
12 KiB
Protocol Buffer
438 lines
12 KiB
Protocol Buffer
syntax = "proto2";
|
|
package hw.trezor.messages.monero;
|
|
|
|
// Sugar for easier handling in Java
|
|
option java_package = "com.satoshilabs.trezor.lib.protobuf";
|
|
option java_outer_classname = "TrezorMessageMonero";
|
|
|
|
/**
|
|
* Request: Ask device for public address derived from seed and address_n
|
|
* @start
|
|
* @next MoneroAddress
|
|
* @next Failure
|
|
*/
|
|
message MoneroGetAddress {
|
|
repeated uint32 address_n = 1; // BIP-32 path to derive the key from master node
|
|
optional bool show_display = 2; // Optionally show on display before sending the result
|
|
optional uint32 network_type = 3; // Main-net / testnet / stagenet
|
|
optional uint32 account = 4; // Major subaddr index
|
|
optional uint32 minor = 5; // Minor subaddr index
|
|
}
|
|
|
|
/**
|
|
* Response: Contains Monero watch-only credentials derived from device private seed
|
|
* @end
|
|
*/
|
|
message MoneroAddress {
|
|
optional bytes address = 1;
|
|
}
|
|
|
|
/**
|
|
* Request: Ask device for watch only credentials
|
|
* @start
|
|
* @next MoneroWatchKey
|
|
* @next Failure
|
|
*/
|
|
message MoneroGetWatchKey {
|
|
repeated uint32 address_n = 1; // BIP-32 path to derive the key from master node
|
|
optional uint32 network_type = 2; // Main-net / testnet / stagenet
|
|
}
|
|
|
|
/**
|
|
* Response: Contains Monero watch-only credentials derived from device private seed
|
|
* @end
|
|
*/
|
|
message MoneroWatchKey {
|
|
optional bytes watch_key = 1;
|
|
optional bytes address = 2;
|
|
}
|
|
|
|
/**
|
|
* Structure representing Monero transaction destination entry
|
|
*/
|
|
message MoneroTransactionDestinationEntry {
|
|
optional uint64 amount = 1;
|
|
optional MoneroAccountPublicAddress addr = 2;
|
|
optional bool is_subaddress = 3;
|
|
|
|
/**
|
|
* Structure representing Monero public address
|
|
*/
|
|
message MoneroAccountPublicAddress {
|
|
optional bytes spend_public_key = 1;
|
|
optional bytes view_public_key = 2;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroTransactionSign. Initializes transaction signing.
|
|
* @start
|
|
* @next MoneroTransactionInitAck
|
|
*/
|
|
message MoneroTransactionInitRequest {
|
|
optional uint32 version = 1;
|
|
repeated uint32 address_n = 2;
|
|
optional uint32 network_type = 3; // Main-net / testnet / stagenet
|
|
optional MoneroTransactionData tsx_data = 4;
|
|
/**
|
|
* Structure representing Monero initial transaction information
|
|
*/
|
|
message MoneroTransactionData {
|
|
optional uint32 version = 1;
|
|
optional bytes payment_id = 2;
|
|
optional uint64 unlock_time = 3;
|
|
repeated MoneroTransactionDestinationEntry outputs = 4;
|
|
optional MoneroTransactionDestinationEntry change_dts = 5;
|
|
optional uint32 num_inputs = 6;
|
|
optional uint32 mixin = 7;
|
|
optional uint64 fee = 8;
|
|
optional uint32 account = 9;
|
|
repeated uint32 minor_indices = 10;
|
|
optional bool is_multisig = 11;
|
|
optional bytes exp_tx_prefix_hash = 12;
|
|
repeated bytes use_tx_keys = 13;
|
|
optional bool is_bulletproof = 14;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Response: Response to transaction signing initialization.
|
|
* @next MoneroTransactionSignRequest
|
|
*/
|
|
message MoneroTransactionInitAck {
|
|
optional uint32 version = 1;
|
|
optional uint32 status = 2;
|
|
optional bool in_memory = 3;
|
|
repeated bytes hmacs = 4;
|
|
optional bool many_inputs = 5;
|
|
optional bool many_outputs = 6;
|
|
}
|
|
|
|
/**
|
|
* Structure representing Monero transaction source entry, UTXO
|
|
*/
|
|
message MoneroTransactionSourceEntry {
|
|
repeated MoneroOutputEntry outputs = 1;
|
|
optional uint64 real_output = 2;
|
|
optional bytes real_out_tx_key = 3;
|
|
repeated bytes real_out_additional_tx_keys = 4;
|
|
optional uint64 real_output_in_tx_index = 5;
|
|
optional uint64 amount = 6;
|
|
optional bool rct = 7;
|
|
optional bytes mask = 8;
|
|
optional MoneroMultisigKLRki multisig_kLRki = 9;
|
|
|
|
message MoneroRctKey {
|
|
optional bytes dest = 1;
|
|
optional bytes mask = 2;
|
|
}
|
|
|
|
message MoneroOutputEntry {
|
|
optional uint64 idx = 1;
|
|
optional MoneroRctKey key = 2;
|
|
}
|
|
|
|
message MoneroMultisigKLRki {
|
|
optional bytes K = 1;
|
|
optional bytes L = 2;
|
|
optional bytes R = 3;
|
|
optional bytes ki = 4;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroTransactionSign. Sends one UTXO to device
|
|
* @next MoneroTransactionSetInputAck
|
|
*/
|
|
message MoneroTransactionSetInputRequest {
|
|
optional uint32 version = 1;
|
|
optional MoneroTransactionSourceEntry src_entr = 2;
|
|
}
|
|
|
|
/**
|
|
* Response: Response to setting UTXO for signature. Contains sealed values needed for further protocol steps.
|
|
* @next MoneroTransactionSignRequest
|
|
*/
|
|
message MoneroTransactionSetInputAck {
|
|
optional bytes vini = 1; // xmrtypes.TxinToKey
|
|
optional bytes vini_hmac = 2;
|
|
optional bytes pseudo_out = 3;
|
|
optional bytes pseudo_out_hmac = 4;
|
|
optional bytes alpha_enc = 5;
|
|
optional bytes spend_enc = 6;
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroTransactionSign. Permutation on key images.
|
|
* @next MoneroTransactionInputsPermutationAck
|
|
*/
|
|
message MoneroTransactionInputsPermutationRequest {
|
|
repeated uint32 perm = 1;
|
|
}
|
|
|
|
/**
|
|
* Response: Response to setting permutation on key images
|
|
* @next MoneroTransactionSignRequest
|
|
*/
|
|
message MoneroTransactionInputsPermutationAck {
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroTransactionSign. Sends one UTXO to device together with sealed values.
|
|
* @next MoneroTransactionInputViniAck
|
|
*/
|
|
message MoneroTransactionInputViniRequest {
|
|
optional MoneroTransactionSourceEntry src_entr = 1;
|
|
optional bytes vini = 2; // xmrtypes.TxinToKey
|
|
optional bytes vini_hmac = 3;
|
|
optional bytes pseudo_out = 4;
|
|
optional bytes pseudo_out_hmac = 5;
|
|
}
|
|
|
|
/**
|
|
* Response: Response to setting UTXO to the device
|
|
* @next MoneroTransactionSignRequest
|
|
*/
|
|
message MoneroTransactionInputViniAck {
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroTransactionSign. Sends one transaction destination to device (HMACed)
|
|
* @next MoneroTransactionSetOutputAck
|
|
*/
|
|
message MoneroTransactionSetOutputRequest {
|
|
optional MoneroTransactionDestinationEntry dst_entr = 1;
|
|
optional bytes dst_entr_hmac = 2;
|
|
}
|
|
|
|
/**
|
|
* Response: Response to setting transaction destination. Contains sealed values needed for further protocol steps.
|
|
* @next MoneroTransactionSignRequest
|
|
*/
|
|
message MoneroTransactionSetOutputAck {
|
|
optional bytes tx_out = 1; // xmrtypes.TxOut
|
|
optional bytes vouti_hmac = 2;
|
|
optional bytes rsig = 3; // byte-encoded range signature
|
|
optional bytes out_pk = 4;
|
|
optional bytes ecdh_info = 5;
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroTransactionSign. Sent after all outputs are sent.
|
|
* @next MoneroTransactionAllOutSetAck
|
|
*/
|
|
message MoneroTransactionAllOutSetRequest {
|
|
}
|
|
|
|
/**
|
|
* Response: After all outputs are sent the initial RCT signature fields are sent.
|
|
* @next MoneroTransactionSignRequest
|
|
*/
|
|
message MoneroTransactionAllOutSetAck {
|
|
optional bytes extra = 1;
|
|
optional bytes tx_prefix_hash = 2;
|
|
optional MoneroRingCtSig rv = 3; // xmrtypes.RctSig
|
|
/*
|
|
* Structure represents initial fields of the Monero RCT signature
|
|
*/
|
|
message MoneroRingCtSig {
|
|
optional uint64 txn_fee = 1;
|
|
optional bytes message = 2;
|
|
optional uint32 rv_type = 3;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroTransactionSign.
|
|
* @next MoneroTransactionMlsagDoneAck
|
|
*/
|
|
message MoneroTransactionMlsagDoneRequest {
|
|
}
|
|
|
|
/**
|
|
* Response: Contains full message hash needed for the signature
|
|
* @next MoneroTransactionSignRequest
|
|
*/
|
|
message MoneroTransactionMlsagDoneAck {
|
|
optional bytes full_message_hash = 1;
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroTransactionSign. Sends UTXO for the signing.
|
|
* @next MoneroTransactionSignInputAck
|
|
*/
|
|
message MoneroTransactionSignInputRequest {
|
|
optional MoneroTransactionSourceEntry src_entr = 1;
|
|
optional bytes vini = 2; // xmrtypes.TxinToKey
|
|
optional bytes vini_hmac = 3;
|
|
optional bytes pseudo_out = 4;
|
|
optional bytes pseudo_out_hmac = 5;
|
|
optional bytes alpha_enc = 6;
|
|
optional bytes spend_enc = 7;
|
|
}
|
|
|
|
/**
|
|
* Response: Contains full MG signature of the UTXO + multisig data if applicable.
|
|
* @next MoneroTransactionSignRequest
|
|
*/
|
|
message MoneroTransactionSignInputAck {
|
|
optional bytes signature = 1;
|
|
optional bytes cout = 2;
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroTransactionSign. Final message of the procol after all UTXOs are signed
|
|
* @next MoneroTransactionFinalAck
|
|
*/
|
|
message MoneroTransactionFinalRequest {
|
|
}
|
|
|
|
/**
|
|
* Response: Contains transaction metadata and encryption keys needed for further transaction operations (e.g. multisig, send proof).
|
|
* @end
|
|
*/
|
|
message MoneroTransactionFinalAck {
|
|
optional bytes cout_key = 1;
|
|
optional bytes salt = 2;
|
|
optional bytes rand_mult = 3;
|
|
optional bytes tx_enc_keys = 4;
|
|
}
|
|
|
|
/**
|
|
* Request: Wrapping request for transaction signature protocol.
|
|
* @wrap MoneroTransactionInitRequest
|
|
* @wrap MoneroTransactionSetInputRequest
|
|
* @wrap MoneroTransactionInputsPermutationRequest
|
|
* @wrap MoneroTransactionInputViniRequest
|
|
* @wrap MoneroTransactionSetOutputRequest
|
|
* @wrap MoneroTransactionAllOutSetRequest
|
|
* @wrap MoneroTransactionMlsagDoneRequest
|
|
* @wrap MoneroTransactionSignInputRequest
|
|
* @wrap MoneroTransactionFinalRequest
|
|
*/
|
|
message MoneroTransactionSignRequest {
|
|
optional MoneroTransactionInitRequest init = 1;
|
|
optional MoneroTransactionSetInputRequest set_input = 2;
|
|
optional MoneroTransactionInputsPermutationRequest input_permutation = 3;
|
|
optional MoneroTransactionInputViniRequest input_vini = 4;
|
|
optional MoneroTransactionSetOutputRequest set_output = 5;
|
|
optional MoneroTransactionAllOutSetRequest all_out_set = 6;
|
|
optional MoneroTransactionMlsagDoneRequest mlsag_done = 7;
|
|
optional MoneroTransactionSignInputRequest sign_input = 8;
|
|
optional MoneroTransactionFinalRequest final_msg = 9;
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroKeyImageSync. Initializing key image sync.
|
|
* @start
|
|
* @next MoneroKeyImageExportInitAck
|
|
*/
|
|
message MoneroKeyImageExportInitRequest {
|
|
optional uint64 num = 1;
|
|
optional bytes hash = 2;
|
|
repeated uint32 address_n = 3; // BIP-32 path to derive the key from master node
|
|
optional uint32 network_type = 4; // Main-net / testnet / stagenet
|
|
repeated MoneroSubAddressIndicesList subs = 5;
|
|
/**
|
|
* Structure representing Monero list of sub-addresses
|
|
*/
|
|
message MoneroSubAddressIndicesList {
|
|
optional uint32 account = 1;
|
|
repeated uint32 minor_indices = 2;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Response: Response to key image sync initialization.
|
|
* @next MoneroKeyImageSyncRequest
|
|
*/
|
|
message MoneroKeyImageExportInitAck {
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroKeyImageSync. Contains batch of the UTXO to export key image for.
|
|
* @next MoneroKeyImageSyncStepAck
|
|
*/
|
|
message MoneroKeyImageSyncStepRequest {
|
|
repeated MoneroTransferDetails tdis = 1;
|
|
/**
|
|
* Structure representing Monero UTXO for key image sync
|
|
*/
|
|
message MoneroTransferDetails {
|
|
optional bytes out_key = 1;
|
|
optional bytes tx_pub_key = 2;
|
|
repeated bytes additional_tx_pub_keys = 3;
|
|
optional uint64 internal_output_index = 4;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Response: Response to key image sync step. Contains encrypted exported key image.
|
|
* @next MoneroKeyImageSyncRequest
|
|
*/
|
|
message MoneroKeyImageSyncStepAck {
|
|
repeated MoneroExportedKeyImage kis = 1;
|
|
/**
|
|
* Structure representing Monero encrypted exported key image
|
|
*/
|
|
message MoneroExportedKeyImage {
|
|
optional bytes iv = 1;
|
|
optional bytes tag = 2;
|
|
optional bytes blob = 3;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Request: Sub request of MoneroKeyImageSync. Final message of the sync protocol.
|
|
* @next MoneroKeyImageSyncFinalAck
|
|
*/
|
|
message MoneroKeyImageSyncFinalRequest {
|
|
}
|
|
|
|
/**
|
|
* Response: Response to key image sync step. Contains encryption keys for exported key images.
|
|
* @end
|
|
*/
|
|
message MoneroKeyImageSyncFinalAck {
|
|
optional bytes enc_key = 1;
|
|
}
|
|
|
|
/**
|
|
* Request: Wrapping request for key image sync protocol.
|
|
* @wrap MoneroKeyImageExportInitRequest
|
|
* @wrap MoneroKeyImageSyncStepRequest
|
|
* @wrap MoneroKeyImageSyncFinalRequest
|
|
*/
|
|
message MoneroKeyImageSyncRequest {
|
|
optional MoneroKeyImageExportInitRequest init = 1;
|
|
optional MoneroKeyImageSyncStepRequest step = 2;
|
|
optional MoneroKeyImageSyncFinalRequest final_msg = 3;
|
|
}
|
|
|
|
/**
|
|
* Request: Universal Monero protocol implementation diagnosis request.
|
|
* @start
|
|
* @next DebugMoneroDiagAck
|
|
*/
|
|
message DebugMoneroDiagRequest {
|
|
optional uint64 ins = 1;
|
|
optional uint64 p1 = 2;
|
|
optional uint64 p2 = 3;
|
|
repeated uint64 pd = 4;
|
|
optional bytes data1 = 5;
|
|
optional bytes data2 = 6;
|
|
}
|
|
|
|
/**
|
|
* Response: Response to Monero diagnosis protocol.
|
|
* @end
|
|
*/
|
|
message DebugMoneroDiagAck {
|
|
optional uint64 ins = 1;
|
|
optional uint64 p1 = 2;
|
|
optional uint64 p2 = 3;
|
|
repeated uint64 pd = 4;
|
|
optional bytes data1 = 5;
|
|
optional bytes data2 = 6;
|
|
}
|