mirror of
https://github.com/trezor/trezor-firmware.git
synced 2025-01-06 21:40:56 +00:00
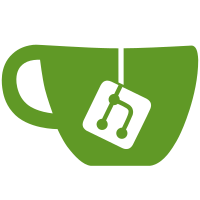
- build variables, lincoln UI selected for compilation only with `UI_LINCOLN_DEV=1` - lincoln directory structure - bootloader UI and assets copied from mercury - FirmwareUI trait functions are empty - Python layout functions are copied from mercury except some of more complicated ones which raise NotImplemented for now
51 lines
1.6 KiB
Python
51 lines
1.6 KiB
Python
from hashlib import blake2s
|
|
|
|
import pytest
|
|
|
|
from trezorlib import firmware, models
|
|
from trezorlib.debuglink import TrezorClientDebugLink as Client
|
|
|
|
# size of FIRMWARE_AREA, see core/embed/models/model_*_layout.c
|
|
FIRMWARE_LENGTHS = {
|
|
models.T1B1: 7 * 128 * 1024 + 64 * 1024,
|
|
models.T2T1: 13 * 128 * 1024,
|
|
models.T2B1: 13 * 128 * 1024,
|
|
models.T3T1: 208 * 8 * 1024,
|
|
models.T3B1: 208 * 8 * 1024,
|
|
models.T3W1: 208 * 8 * 1024, # FIXME: fill in the correct value
|
|
}
|
|
|
|
|
|
def test_firmware_hash_emu(client: Client) -> None:
|
|
if client.features.fw_vendor != "EMULATOR":
|
|
pytest.skip("Only for emulator")
|
|
|
|
data = b"\xff" * FIRMWARE_LENGTHS[client.model]
|
|
|
|
expected_hash = blake2s(data).digest()
|
|
hash = firmware.get_hash(client, None)
|
|
assert hash == expected_hash
|
|
|
|
challenge = b"Hello Trezor"
|
|
expected_hash = blake2s(data, key=challenge).digest()
|
|
hash = firmware.get_hash(client, challenge)
|
|
assert hash == expected_hash
|
|
|
|
|
|
def test_firmware_hash_hw(client: Client) -> None:
|
|
if client.features.fw_vendor == "EMULATOR":
|
|
pytest.skip("Only for hardware")
|
|
|
|
# TODO get firmware image from outside the environment, check for actual result
|
|
challenge = b"Hello Trezor"
|
|
empty_data = b"\xff" * FIRMWARE_LENGTHS[client.model]
|
|
empty_hash = blake2s(empty_data).digest()
|
|
empty_hash_challenge = blake2s(empty_data, key=challenge).digest()
|
|
|
|
hash = firmware.get_hash(client, None)
|
|
assert hash != empty_hash
|
|
|
|
hash2 = firmware.get_hash(client, challenge)
|
|
assert hash != hash2
|
|
assert hash2 != empty_hash_challenge
|