mirror of
https://github.com/pi-hole/pi-hole
synced 2024-11-18 06:08:21 +00:00
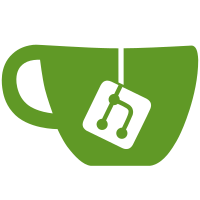
* Fix handling of wildcard help text * Rewrite help text for better handling of params * Replace misleading letter variable * stash changes on branch switch, else it fails if any changes have been made. * Make changes according to comment in #1384 * Update queryFunc() * Allow scanList() to search files using a wildcard by removing quotes wrapped around `${list}` * scanList() will not provide a domain ouput on each string if exact is specified (`grep -l`) * Remove unused processWildcards() function * Return a message if no domain is specified * IDN domains are converted to punycode when running a `pihole -q` search if the `python` package is available, otherwise will revert to current behaviour * Scan Blacklist & Wildcards first, exiting from search if a match is found (Fixes #1330) * Use one `grep` subshell to search for all "*.domains" lists at once (opposed to looping to get every matching file name, and then spawning a `grep` instance for every matching file) * queryFunc() will not return "(0 results)" output from files where no match is found * Sort results based off list number * Return a message if no results are found * Update basic-install.sh * Update block page. Allow for setupVars setting of CUSTOMBLOCKPAGE (bool) to prevent it being overwritten * simplify * further simplify * fix inteliJ IDEA complaints * even further simplify * tidy up output * revert line, looks tidyer * clarify * Revert "Ensure any changes to blocking page are updated." * We test for dpkg lock on line 830 directly, no need for the check also in the template section. Signed-off-by: Dan Schaper <dan.schaper@pi-hole.net> * Display FTL version & version.sh rewrite While testing to make sure `pihole -v` would output `pihole-FTL version`, I noticed some options didn't work how I expected them to. For example, if I use `pihole -v -p`, I would expect to see the version output of Pi-hole Core. Instead, I'm informed that it's an invalid option. I've had the following things in mind while rewriting this: * I'm operating under the assumption that FTL is only installed if the Admin Console is (Line 113 exit 0) * I have modified the help text to only output with `pihole -v --help` * I have modified all output to be more similar to the output style of `grep` and `curl` (Ditching ":::") Testing output: ``` w3k@MCT:~$ pihole -v Pi-hole version is v3.0.1-14-ga928cd3 (Latest: v3.0.1) Admin Console version is v3.0-9-g3760482 (Latest: v3.0.1) FTL version is v2.6.2 (Latest: v2.6.2) w3k@MCT:~$ pihole -v -c Current Pi-hole version is v3.0.1-14-ga928cd3 Current Admin Console version is v3.0-9-g3760482 Current FTL version is v2.6.2 w3k@MCT:~$ pihole -v -l Latest Pi-hole version is v3.0.1 Latest Admin Console version is v3.0.1 Latest FTL version is v2.6.2 w3k@MCT:~$ pihole -v -p --hash Current Pi-hole hash isa928cd3
w3k@MCT:~$ pihole -v -a --hash Current Admin Console hash is 3760482 w3k@MCT:~$ pihole -v --help Usage: pihole -v [REPO | OPTION] [OPTION] Show Pi-hole, Web Admin & FTL versions <Shows all Repositories and Options> w3k@MCT:~$ pihole -v -foo Invalid Option! ``` * Update -h to work as --hash Also provide error output as per https://github.com/pi-hole/pi-hole/pull/1447#issuecomment-300600093 * Perform EXACT searches on HOSTS lists correctly `\s` on the end may be overkill, but it is the existing scanList() behaviour. * Fixed indentation * Minimise string duplication & other minor changes Instead of duplicating output strings, rewrite core/web/ftlOutput() into one neat versionOutput(). * Modified syntax to be valid for Shellcheck * Log and echo gateway responses * Update queryFunc() to search Whitelist If there is a match in Whitelist/Blacklist/Wildcards, `[ ! -t 1 ]` will cause the search to end if the terminal is closed when the script is called. This has the intended effect of allowing a user to search for a W/B/W domain (as well as all the adlists it's found in) using `pihole -q` via Terminal, but the script will stop searching after a W/B/W match when called by the block page. * Wrap in double brackets * Provide remote hashes for version.sh * Provide remote hashes for comparison * Use double braces for all conditions (for consistency) * Suppress potential "cd" error output * Provide "not applicable" output upon any hash request for FTL * whitelist on website blocked doesnt work (#1452) Since Pi-hole redirects ad domains to itself, accessing the script via de.ign.com is the same as pi.hole in this case. The fix should be as simple as adding a / before admin on this line. * Solve piholeLogFlush.sh having to be issued 2 x to clear logs (#1460) Simplified the command -v syntax, and added a sleep 3 timer to the first execution of the log rotation. The second execution was being issued while the first was still running, thus it would fail and you would have to issue the "Flush Logs" command a second time. * Use `echo "ABC" | pihole tricorder` to upload to Pi-hole's medical tricorder. Uses SSL if available. * Update list.sh I believe this has feature parity with `sed /foo/ Id` but also supports busybox, and my alpine docker ;) * Document `sed` substitution for user readability Comment the oneliner with explanations of what each step does. * Update Help Output (#1467) * File consistency * Tabs to 2 spaces * Corrected indenting * Double braced conditionals * Quoted variables within conditionals * Standardise core help text * Added help text for disable command * Added help text for logging command * Clean up * Fixed certain new lines and spaces * Sync with development branch * Formatting consistency * Tabs to 2 spaces * Corrected indenting * Double braced conditionals * Quoted variables within conditionals * Fixed certain newlines and spaces * Admin help text * Added help text for interface command * Sync with development branch * Formatting consistency * Tabs to 2 spaces * Fixed some wording * Fixed certain spaces * Formatting consistency * Minor wording changes * Tabs to 2 spaces * Corrected indenting * Double braced conditionals * Quoted variables within conditionals * Fixed certain newlines and spaces * Blacklist help text * Formatting consistency * Tabs to 2 spaces * Corrected indenting * Cronometer help text * Formatting consistency * Fixed certain newlines and spaces * Corrected indenting * Checkout warning alteration * Add checkout help text * Corrected help output * Show help for "pihole -a -i --help" * Fix "pihole disable --help" and "pihole -l --help" * Show help for "pihole -v -h" * Indent output text * Minor help text change * Show help for "pihole checkout --help" * Tricorder: Insecure Opt-out * Check to see if Tricorder is being called directly * Provide opt-out for insecure transmission of debug log * Remove mention of internal function from help menu * 🌮 is the new :shipit: squirrel * Wording changes and bug fix * Fix wildcard help text * -wild is not a valid option since we're already using -wild * Fix logrotation: manual flushing should be done twice, but automated rotation at midnight should only be done *once*! * Print echos only when manual flushing is requested * Add "quiet" mode + update comments in the cron file * Confirm Tricorder is online * Scan port 9998 to confirm the availability of "tricorder.pi-hole.net" * Exit codes for upload process * Formatting consistency * Add link to Windows DNS Swapper See #1400 * Install loopback firewall rules for FTL (#1419) * Install loopback firewall rules for FTL * FirewallD FTL ports Signed-off-by: Dan Schaper <dan.schaper@pi-hole.net> * Remove firewallD FTL local rules. Local rules should not be blocked in firewallD, not requred for internal service FTD> * Reinstate https rules, and delete FTL rules Fixes earlier commit. * Retrieve local repos on repair (#1481) * Retrieve local repos on repair * Change conditional to check for repair * Change wording of Update/Reconfigure message * Fixed indenting * Perform "git reset --hard" on reconfigure * Change directory before trying to reset repository. Fixes #1489 * No need to `cd $PWD` as it doesn't affect flow of caller script. Signed-off-by: Dan Schaper <dan.schaper@pi-hole.net> * Refine output of password status in basic-install.sh:displayFinalMessage(). Fixes #1488 (#1490) * Rewrite Chronometer to output more stats * Fix output IPv4 addr when removing CIDR notation (#1498) * Move wildcards file if blocking is disabled (#1495) * Move wildcards file if blocking is diabled * Delete newline * Roll back merge #1417 (#1494) * Update ISSUE_TEMPLATE.md * Remove Question option * Prefer ULA over GUA addresses [IPv6] (#1508) * On installs with GUA and ULA's we should prefer ULA's as it's been demonstrated that GUA's can and often are rotated by ISPs. Fixes #1473 * Add test for link-local address detection * Add ULA-only and GUA-only tests * Add test_IPv6_GUA_ULA_test and test_IPv6_ULA_GUA_test * Add "" * Add mock_command_2 command that can mock a command with more than one argument (as "ip -6 address") and result multiple lines of results * Make mock_command_2 more similar to the original mock_command * Correct comments * Fixed remaining comments * Fixed one last comment... * Fixed a comment... * Add weekly logrotation of FTL's log (#1509) * Update LICENSE of the project to EUPL v1.2 * Make clear that NO is the default if the user just hits return (#1514) * Add tricorderFunc back as usable function (#1515) As per #1464 * Don't update FTL when there is a core update (as this will update FTL a second time). Fixes #1516 * Add FTL tests to the test suite (#1510) * Add first version of FTL tests * Wait one second to allow FTL to start up and analyze our mock log * Add test_FTL_telnet_statistics * Added test_FTL_telnet_top_clients * Add test_FTL_telnet_top_domains * Revert "Add FTL tests to the test suite (#1510)" (#1519) This reverts commitcf6a1ac9ad
. * Trim version output when update is successful (#1527) * Change ownership of /etc/pihole to user/group pihole. Fixes #1529 (#1530) * Delete temporary files after installing the FTL binary. Fixes #1525 * Change from admin to approvers teams * Introduce new file black.list for blacklist content * Add "pihole -g -b" to *only* update black.list (saves a bunch of time when adding/changing only blacklisted files - won'tdownload lal lists, but only processes the blacklist and restars dnsmasq) * Remove useless cat * Improve displayed messages and overall logic * Disable black.list on "pihole disable" * cp + rm === mv (well, almost)
466 lines
12 KiB
Bash
Executable File
466 lines
12 KiB
Bash
Executable File
#!/usr/bin/env bash
|
|
# Pi-hole: A black hole for Internet advertisements
|
|
# (c) 2017 Pi-hole, LLC (https://pi-hole.net)
|
|
# Network-wide ad blocking via your own hardware.
|
|
#
|
|
# Web interface settings
|
|
#
|
|
# This file is copyright under the latest version of the EUPL.
|
|
# Please see LICENSE file for your rights under this license.
|
|
|
|
readonly setupVars="/etc/pihole/setupVars.conf"
|
|
readonly dnsmasqconfig="/etc/dnsmasq.d/01-pihole.conf"
|
|
readonly dhcpconfig="/etc/dnsmasq.d/02-pihole-dhcp.conf"
|
|
# 03 -> wildcards
|
|
readonly dhcpstaticconfig="/etc/dnsmasq.d/04-pihole-static-dhcp.conf"
|
|
|
|
helpFunc() {
|
|
echo "Usage: pihole -a [options]
|
|
Example: pihole -a -p password
|
|
Set options for the Admin Console
|
|
|
|
Options:
|
|
-f, flush Flush the Pi-hole log
|
|
-p, password Set Admin Console password
|
|
-c, celsius Set Celsius as preferred temperature unit
|
|
-f, fahrenheit Set Fahrenheit as preferred temperature unit
|
|
-k, kelvin Set Kelvin as preferred temperature unit
|
|
-h, --help Show this help dialog
|
|
-i, interface Specify dnsmasq's interface listening behavior
|
|
Add '-h' for more info on interface usage"
|
|
exit 0
|
|
}
|
|
|
|
add_setting() {
|
|
echo "${1}=${2}" >> "${setupVars}"
|
|
}
|
|
|
|
delete_setting() {
|
|
sed -i "/${1}/d" "${setupVars}"
|
|
}
|
|
|
|
change_setting() {
|
|
delete_setting "${1}"
|
|
add_setting "${1}" "${2}"
|
|
}
|
|
|
|
add_dnsmasq_setting() {
|
|
if [[ "${2}" != "" ]]; then
|
|
echo "${1}=${2}" >> "${dnsmasqconfig}"
|
|
else
|
|
echo "${1}" >> "${dnsmasqconfig}"
|
|
fi
|
|
}
|
|
|
|
delete_dnsmasq_setting() {
|
|
sed -i "/${1}/d" "${dnsmasqconfig}"
|
|
}
|
|
|
|
SetTemperatureUnit() {
|
|
change_setting "TEMPERATUREUNIT" "${unit}"
|
|
}
|
|
|
|
HashPassword() {
|
|
# Compute password hash twice to avoid rainbow table vulnerability
|
|
return=$(echo -n ${1} | sha256sum | sed 's/\s.*$//')
|
|
return=$(echo -n ${return} | sha256sum | sed 's/\s.*$//')
|
|
echo ${return}
|
|
}
|
|
|
|
SetWebPassword() {
|
|
if [ "${SUDO_USER}" == "www-data" ]; then
|
|
echo "Security measure: user www-data is not allowed to change webUI password!"
|
|
echo "Exiting"
|
|
exit 1
|
|
fi
|
|
|
|
if [ "${SUDO_USER}" == "lighttpd" ]; then
|
|
echo "Security measure: user lighttpd is not allowed to change webUI password!"
|
|
echo "Exiting"
|
|
exit 1
|
|
fi
|
|
|
|
if (( ${#args[2]} > 0 )) ; then
|
|
readonly PASSWORD="${args[2]}"
|
|
readonly CONFIRM="${PASSWORD}"
|
|
else
|
|
read -s -p "Enter New Password (Blank for no password): " PASSWORD
|
|
echo ""
|
|
|
|
if [ "${PASSWORD}" == "" ]; then
|
|
change_setting "WEBPASSWORD" ""
|
|
echo "Password Removed"
|
|
exit 0
|
|
fi
|
|
|
|
read -s -p "Confirm Password: " CONFIRM
|
|
echo ""
|
|
fi
|
|
|
|
if [ "${PASSWORD}" == "${CONFIRM}" ] ; then
|
|
hash=$(HashPassword ${PASSWORD})
|
|
# Save hash to file
|
|
change_setting "WEBPASSWORD" "${hash}"
|
|
echo "New password set"
|
|
else
|
|
echo "Passwords don't match. Your password has not been changed"
|
|
exit 1
|
|
fi
|
|
}
|
|
|
|
ProcessDNSSettings() {
|
|
source "${setupVars}"
|
|
|
|
delete_dnsmasq_setting "server"
|
|
|
|
COUNTER=1
|
|
while [[ 1 ]]; do
|
|
var=PIHOLE_DNS_${COUNTER}
|
|
if [ -z "${!var}" ]; then
|
|
break;
|
|
fi
|
|
add_dnsmasq_setting "server" "${!var}"
|
|
let COUNTER=COUNTER+1
|
|
done
|
|
|
|
delete_dnsmasq_setting "domain-needed"
|
|
|
|
if [[ "${DNS_FQDN_REQUIRED}" == true ]]; then
|
|
add_dnsmasq_setting "domain-needed"
|
|
fi
|
|
|
|
delete_dnsmasq_setting "bogus-priv"
|
|
|
|
if [[ "${DNS_BOGUS_PRIV}" == true ]]; then
|
|
add_dnsmasq_setting "bogus-priv"
|
|
fi
|
|
|
|
delete_dnsmasq_setting "dnssec"
|
|
delete_dnsmasq_setting "trust-anchor="
|
|
|
|
if [[ "${DNSSEC}" == true ]]; then
|
|
echo "dnssec
|
|
trust-anchor=.,19036,8,2,49AAC11D7B6F6446702E54A1607371607A1A41855200FD2CE1CDDE32F24E8FB5
|
|
" >> "${dnsmasqconfig}"
|
|
fi
|
|
|
|
delete_dnsmasq_setting "host-record"
|
|
|
|
if [ ! -z "${HOSTRECORD}" ]; then
|
|
add_dnsmasq_setting "host-record" "${HOSTRECORD}"
|
|
fi
|
|
|
|
# Setup interface listening behavior of dnsmasq
|
|
delete_dnsmasq_setting "interface"
|
|
delete_dnsmasq_setting "local-service"
|
|
|
|
if [[ "${DNSMASQ_LISTENING}" == "all" ]]; then
|
|
# Listen on all interfaces, permit all origins
|
|
add_dnsmasq_setting "except-interface" "nonexisting"
|
|
elif [[ "${DNSMASQ_LISTENING}" == "local" ]]; then
|
|
# Listen only on all interfaces, but only local subnets
|
|
add_dnsmasq_setting "local-service"
|
|
else
|
|
# Listen only on one interface
|
|
add_dnsmasq_setting "interface" "${PIHOLE_INTERFACE}"
|
|
fi
|
|
|
|
}
|
|
|
|
SetDNSServers() {
|
|
# Save setting to file
|
|
delete_setting "PIHOLE_DNS"
|
|
IFS=',' read -r -a array <<< "${args[2]}"
|
|
for index in "${!array[@]}"
|
|
do
|
|
add_setting "PIHOLE_DNS_$((index+1))" "${array[index]}"
|
|
done
|
|
|
|
if [[ "${args[3]}" == "domain-needed" ]]; then
|
|
change_setting "DNS_FQDN_REQUIRED" "true"
|
|
else
|
|
change_setting "DNS_FQDN_REQUIRED" "false"
|
|
fi
|
|
|
|
if [[ "${args[4]}" == "bogus-priv" ]]; then
|
|
change_setting "DNS_BOGUS_PRIV" "true"
|
|
else
|
|
change_setting "DNS_BOGUS_PRIV" "false"
|
|
fi
|
|
|
|
if [[ "${args[5]}" == "dnssec" ]]; then
|
|
change_setting "DNSSEC" "true"
|
|
else
|
|
change_setting "DNSSEC" "false"
|
|
fi
|
|
|
|
ProcessDNSSettings
|
|
|
|
# Restart dnsmasq to load new configuration
|
|
RestartDNS
|
|
}
|
|
|
|
SetExcludeDomains() {
|
|
change_setting "API_EXCLUDE_DOMAINS" "${args[2]}"
|
|
}
|
|
|
|
SetExcludeClients() {
|
|
change_setting "API_EXCLUDE_CLIENTS" "${args[2]}"
|
|
}
|
|
|
|
Reboot() {
|
|
nohup bash -c "sleep 5; reboot" &> /dev/null </dev/null &
|
|
}
|
|
|
|
RestartDNS() {
|
|
if [ -x "$(command -v systemctl)" ]; then
|
|
systemctl restart dnsmasq &> /dev/null
|
|
else
|
|
service dnsmasq restart &> /dev/null
|
|
fi
|
|
}
|
|
|
|
SetQueryLogOptions() {
|
|
change_setting "API_QUERY_LOG_SHOW" "${args[2]}"
|
|
}
|
|
|
|
ProcessDHCPSettings() {
|
|
source "${setupVars}"
|
|
|
|
if [[ "${DHCP_ACTIVE}" == "true" ]]; then
|
|
interface=$(grep 'PIHOLE_INTERFACE=' /etc/pihole/setupVars.conf | sed "s/.*=//")
|
|
|
|
# Use eth0 as fallback interface
|
|
if [ -z ${interface} ]; then
|
|
interface="eth0"
|
|
fi
|
|
|
|
if [[ "${PIHOLE_DOMAIN}" == "" ]]; then
|
|
PIHOLE_DOMAIN="local"
|
|
change_setting "PIHOLE_DOMAIN" "${PIHOLE_DOMAIN}"
|
|
fi
|
|
|
|
if [[ "${DHCP_LEASETIME}" == "0" ]]; then
|
|
leasetime="infinite"
|
|
elif [[ "${DHCP_LEASETIME}" == "" ]]; then
|
|
leasetime="24h"
|
|
change_setting "DHCP_LEASETIME" "${leasetime}"
|
|
else
|
|
leasetime="${DHCP_LEASETIME}h"
|
|
fi
|
|
|
|
# Write settings to file
|
|
echo "###############################################################################
|
|
# DHCP SERVER CONFIG FILE AUTOMATICALLY POPULATED BY PI-HOLE WEB INTERFACE. #
|
|
# ANY CHANGES MADE TO THIS FILE WILL BE LOST ON CHANGE #
|
|
###############################################################################
|
|
dhcp-authoritative
|
|
dhcp-range=${DHCP_START},${DHCP_END},${leasetime}
|
|
dhcp-option=option:router,${DHCP_ROUTER}
|
|
dhcp-leasefile=/etc/pihole/dhcp.leases
|
|
#quiet-dhcp
|
|
" > "${dhcpconfig}"
|
|
|
|
if [[ "${PIHOLE_DOMAIN}" != "none" ]]; then
|
|
echo "domain=${PIHOLE_DOMAIN}" >> "${dhcpconfig}"
|
|
fi
|
|
|
|
if [[ "${DHCP_IPv6}" == "true" ]]; then
|
|
echo "#quiet-dhcp6
|
|
#enable-ra
|
|
dhcp-option=option6:dns-server,[::]
|
|
dhcp-range=::100,::1ff,constructor:${interface},ra-names,slaac,${leasetime}
|
|
ra-param=*,0,0
|
|
" >> "${dhcpconfig}"
|
|
fi
|
|
|
|
else
|
|
rm "${dhcpconfig}" &> /dev/null
|
|
fi
|
|
}
|
|
|
|
EnableDHCP() {
|
|
change_setting "DHCP_ACTIVE" "true"
|
|
change_setting "DHCP_START" "${args[2]}"
|
|
change_setting "DHCP_END" "${args[3]}"
|
|
change_setting "DHCP_ROUTER" "${args[4]}"
|
|
change_setting "DHCP_LEASETIME" "${args[5]}"
|
|
change_setting "PIHOLE_DOMAIN" "${args[6]}"
|
|
change_setting "DHCP_IPv6" "${args[7]}"
|
|
|
|
# Remove possible old setting from file
|
|
delete_dnsmasq_setting "dhcp-"
|
|
delete_dnsmasq_setting "quiet-dhcp"
|
|
|
|
ProcessDHCPSettings
|
|
|
|
RestartDNS
|
|
}
|
|
|
|
DisableDHCP() {
|
|
change_setting "DHCP_ACTIVE" "false"
|
|
|
|
# Remove possible old setting from file
|
|
delete_dnsmasq_setting "dhcp-"
|
|
delete_dnsmasq_setting "quiet-dhcp"
|
|
|
|
ProcessDHCPSettings
|
|
|
|
RestartDNS
|
|
}
|
|
|
|
SetWebUILayout() {
|
|
change_setting "WEBUIBOXEDLAYOUT" "${args[2]}"
|
|
}
|
|
|
|
CustomizeAdLists() {
|
|
list="/etc/pihole/adlists.list"
|
|
|
|
if [[ "${args[2]}" == "enable" ]]; then
|
|
sed -i "\\@${args[3]}@s/^#http/http/g" "${list}"
|
|
elif [[ "${args[2]}" == "disable" ]]; then
|
|
sed -i "\\@${args[3]}@s/^http/#http/g" "${list}"
|
|
elif [[ "${args[2]}" == "add" ]]; then
|
|
echo "${args[3]}" >> ${list}
|
|
elif [[ "${args[2]}" == "del" ]]; then
|
|
var=$(echo "${args[3]}" | sed 's/\//\\\//g')
|
|
sed -i "/${var}/Id" "${list}"
|
|
else
|
|
echo "Not permitted"
|
|
return 1
|
|
fi
|
|
}
|
|
|
|
SetPrivacyMode() {
|
|
if [[ "${args[2]}" == "true" ]]; then
|
|
change_setting "API_PRIVACY_MODE" "true"
|
|
else
|
|
change_setting "API_PRIVACY_MODE" "false"
|
|
fi
|
|
}
|
|
|
|
ResolutionSettings() {
|
|
typ="${args[2]}"
|
|
state="${args[3]}"
|
|
|
|
if [[ "${typ}" == "forward" ]]; then
|
|
change_setting "API_GET_UPSTREAM_DNS_HOSTNAME" "${state}"
|
|
elif [[ "${typ}" == "clients" ]]; then
|
|
change_setting "API_GET_CLIENT_HOSTNAME" "${state}"
|
|
fi
|
|
}
|
|
|
|
AddDHCPStaticAddress() {
|
|
mac="${args[2]}"
|
|
ip="${args[3]}"
|
|
host="${args[4]}"
|
|
|
|
if [[ "${ip}" == "noip" ]]; then
|
|
# Static host name
|
|
echo "dhcp-host=${mac},${host}" >> "${dhcpstaticconfig}"
|
|
elif [[ "${host}" == "nohost" ]]; then
|
|
# Static IP
|
|
echo "dhcp-host=${mac},${ip}" >> "${dhcpstaticconfig}"
|
|
else
|
|
# Full info given
|
|
echo "dhcp-host=${mac},${ip},${host}" >> "${dhcpstaticconfig}"
|
|
fi
|
|
}
|
|
|
|
RemoveDHCPStaticAddress() {
|
|
mac="${args[2]}"
|
|
sed -i "/dhcp-host=${mac}.*/d" "${dhcpstaticconfig}"
|
|
}
|
|
|
|
SetHostRecord() {
|
|
if [ -n "${args[3]}" ]; then
|
|
change_setting "HOSTRECORD" "${args[2]},${args[3]}"
|
|
echo "Setting host record for ${args[2]} -> ${args[3]}"
|
|
else
|
|
change_setting "HOSTRECORD" ""
|
|
echo "Removing host record"
|
|
fi
|
|
|
|
ProcessDNSSettings
|
|
|
|
# Restart dnsmasq to load new configuration
|
|
RestartDNS
|
|
}
|
|
|
|
SetListeningMode() {
|
|
source "${setupVars}"
|
|
|
|
if [[ "$3" == "-h" ]] || [[ "$3" == "--help" ]]; then
|
|
echo "Usage: pihole -a -i [interface]
|
|
Example: 'pihole -a -i local'
|
|
Specify dnsmasq's network interface listening behavior
|
|
|
|
Interfaces:
|
|
local Listen on all interfaces, but only allow queries from
|
|
devices that are at most one hop away (local devices)
|
|
single Listen only on ${PIHOLE_INTERFACE} interface
|
|
all Listen on all interfaces, permit all origins"
|
|
exit 0
|
|
fi
|
|
|
|
if [[ "${args[2]}" == "all" ]]; then
|
|
echo "Listening on all interfaces, permiting all origins, hope you have a firewall!"
|
|
change_setting "DNSMASQ_LISTENING" "all"
|
|
elif [[ "${args[2]}" == "local" ]]; then
|
|
echo "Listening on all interfaces, permitting only origins that are at most one hop away (local devices)"
|
|
change_setting "DNSMASQ_LISTENING" "local"
|
|
else
|
|
echo "Listening only on interface ${PIHOLE_INTERFACE}"
|
|
change_setting "DNSMASQ_LISTENING" "single"
|
|
fi
|
|
|
|
# Don't restart DNS server yet because other settings
|
|
# will be applied afterwards if "-web" is set
|
|
if [[ "${args[3]}" != "-web" ]]; then
|
|
ProcessDNSSettings
|
|
# Restart dnsmasq to load new configuration
|
|
RestartDNS
|
|
fi
|
|
}
|
|
|
|
Teleporter() {
|
|
local datetimestamp=$(date "+%Y-%m-%d_%H-%M-%S")
|
|
php /var/www/html/admin/scripts/pi-hole/php/teleporter.php > "pi-hole-teleporter_${datetimestamp}.zip"
|
|
}
|
|
|
|
main() {
|
|
args=("$@")
|
|
|
|
case "${args[1]}" in
|
|
"-p" | "password" ) SetWebPassword;;
|
|
"-c" | "celsius" ) unit="C"; SetTemperatureUnit;;
|
|
"-f" | "fahrenheit" ) unit="F"; SetTemperatureUnit;;
|
|
"-k" | "kelvin" ) unit="K"; SetTemperatureUnit;;
|
|
"setdns" ) SetDNSServers;;
|
|
"setexcludedomains" ) SetExcludeDomains;;
|
|
"setexcludeclients" ) SetExcludeClients;;
|
|
"reboot" ) Reboot;;
|
|
"restartdns" ) RestartDNS;;
|
|
"setquerylog" ) SetQueryLogOptions;;
|
|
"enabledhcp" ) EnableDHCP;;
|
|
"disabledhcp" ) DisableDHCP;;
|
|
"layout" ) SetWebUILayout;;
|
|
"-h" | "--help" ) helpFunc;;
|
|
"privacymode" ) SetPrivacyMode;;
|
|
"resolve" ) ResolutionSettings;;
|
|
"addstaticdhcp" ) AddDHCPStaticAddress;;
|
|
"removestaticdhcp" ) RemoveDHCPStaticAddress;;
|
|
"hostrecord" ) SetHostRecord;;
|
|
"-i" | "interface" ) SetListeningMode "$@";;
|
|
"-t" | "teleporter" ) Teleporter;;
|
|
"adlist" ) CustomizeAdLists;;
|
|
* ) helpFunc;;
|
|
esac
|
|
|
|
shift
|
|
|
|
if [[ $# = 0 ]]; then
|
|
helpFunc
|
|
fi
|
|
}
|