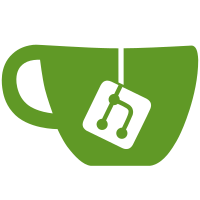
When an attacker uses a <form> to downvote a comment, the browser *should* add a `Content-Type: ...` header with three possible values: * application/x-www-form-urlencoded * multipart/form-data * text/plain If the header is not sent or requests `application/json`, the request is not forged (XHR is restricted by CORS separately).
40 lines
783 B
Python
40 lines
783 B
Python
# -*- encoding: utf-8 -*-
|
|
|
|
import json
|
|
|
|
from werkzeug.test import Client
|
|
|
|
class FakeIP(object):
|
|
|
|
def __init__(self, app, ip):
|
|
self.app = app
|
|
self.ip = ip
|
|
|
|
def __call__(self, environ, start_response):
|
|
environ['REMOTE_ADDR'] = self.ip
|
|
return self.app(environ, start_response)
|
|
|
|
|
|
class JSONClient(Client):
|
|
|
|
def open(self, *args, **kwargs):
|
|
kwargs.setdefault('content_type', 'application/json')
|
|
return super(JSONClient, self).open(*args, **kwargs)
|
|
|
|
|
|
class Dummy:
|
|
|
|
status = 200
|
|
|
|
def __enter__(self):
|
|
return self
|
|
|
|
def read(self):
|
|
return ''
|
|
|
|
def __exit__(self, exc_type, exc_val, exc_tb):
|
|
pass
|
|
|
|
curl = lambda method, host, path: Dummy()
|
|
loads = lambda data: json.loads(data.decode('utf-8'))
|