mirror of
https://github.com/hashcat/hashcat.git
synced 2024-11-02 05:30:19 +00:00
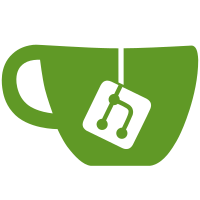
The 7zip, scrypt and stdout kernels differ from the others in their function declarations somewhat. Unify them and substitute with macros. Also remove a few superfluous (bogus) consts which were introduced in the previous PR.
233 lines
5.5 KiB
Common Lisp
233 lines
5.5 KiB
Common Lisp
/**
|
|
* Author......: See docs/credits.txt
|
|
* License.....: MIT
|
|
*/
|
|
|
|
#define NEW_SIMD_CODE
|
|
|
|
#include "inc_vendor.cl"
|
|
#include "inc_hash_constants.h"
|
|
#include "inc_hash_functions.cl"
|
|
#include "inc_types.cl"
|
|
#include "inc_common.cl"
|
|
#include "inc_simd.cl"
|
|
#include "inc_hash_sha1.cl"
|
|
|
|
#define COMPARE_S "inc_comp_single.cl"
|
|
#define COMPARE_M "inc_comp_multi.cl"
|
|
|
|
DECLSPEC void hmac_sha1_run_V (u32x *w0, u32x *w1, u32x *w2, u32x *w3, u32x *ipad, u32x *opad, u32x *digest)
|
|
{
|
|
digest[0] = ipad[0];
|
|
digest[1] = ipad[1];
|
|
digest[2] = ipad[2];
|
|
digest[3] = ipad[3];
|
|
digest[4] = ipad[4];
|
|
|
|
sha1_transform_vector (w0, w1, w2, w3, digest);
|
|
|
|
w0[0] = digest[0];
|
|
w0[1] = digest[1];
|
|
w0[2] = digest[2];
|
|
w0[3] = digest[3];
|
|
w1[0] = digest[4];
|
|
w1[1] = 0x80000000;
|
|
w1[2] = 0;
|
|
w1[3] = 0;
|
|
w2[0] = 0;
|
|
w2[1] = 0;
|
|
w2[2] = 0;
|
|
w2[3] = 0;
|
|
w3[0] = 0;
|
|
w3[1] = 0;
|
|
w3[2] = 0;
|
|
w3[3] = (64 + 20) * 8;
|
|
|
|
digest[0] = opad[0];
|
|
digest[1] = opad[1];
|
|
digest[2] = opad[2];
|
|
digest[3] = opad[3];
|
|
digest[4] = opad[4];
|
|
|
|
sha1_transform_vector (w0, w1, w2, w3, digest);
|
|
}
|
|
|
|
__kernel void m12000_init (KERN_ATTR_TMPS_ESALT (pbkdf2_sha1_tmp_t, pbkdf2_sha1_t))
|
|
{
|
|
/**
|
|
* base
|
|
*/
|
|
|
|
const u64 gid = get_global_id (0);
|
|
|
|
if (gid >= gid_max) return;
|
|
|
|
sha1_hmac_ctx_t sha1_hmac_ctx;
|
|
|
|
sha1_hmac_init_global_swap (&sha1_hmac_ctx, pws[gid].i, pws[gid].pw_len);
|
|
|
|
tmps[gid].ipad[0] = sha1_hmac_ctx.ipad.h[0];
|
|
tmps[gid].ipad[1] = sha1_hmac_ctx.ipad.h[1];
|
|
tmps[gid].ipad[2] = sha1_hmac_ctx.ipad.h[2];
|
|
tmps[gid].ipad[3] = sha1_hmac_ctx.ipad.h[3];
|
|
tmps[gid].ipad[4] = sha1_hmac_ctx.ipad.h[4];
|
|
|
|
tmps[gid].opad[0] = sha1_hmac_ctx.opad.h[0];
|
|
tmps[gid].opad[1] = sha1_hmac_ctx.opad.h[1];
|
|
tmps[gid].opad[2] = sha1_hmac_ctx.opad.h[2];
|
|
tmps[gid].opad[3] = sha1_hmac_ctx.opad.h[3];
|
|
tmps[gid].opad[4] = sha1_hmac_ctx.opad.h[4];
|
|
|
|
sha1_hmac_update_global_swap (&sha1_hmac_ctx, esalt_bufs[digests_offset].salt_buf, salt_bufs[salt_pos].salt_len);
|
|
|
|
for (u32 i = 0, j = 1; i < 4; i += 5, j += 1)
|
|
{
|
|
sha1_hmac_ctx_t sha1_hmac_ctx2 = sha1_hmac_ctx;
|
|
|
|
u32 w0[4];
|
|
u32 w1[4];
|
|
u32 w2[4];
|
|
u32 w3[4];
|
|
|
|
w0[0] = j;
|
|
w0[1] = 0;
|
|
w0[2] = 0;
|
|
w0[3] = 0;
|
|
w1[0] = 0;
|
|
w1[1] = 0;
|
|
w1[2] = 0;
|
|
w1[3] = 0;
|
|
w2[0] = 0;
|
|
w2[1] = 0;
|
|
w2[2] = 0;
|
|
w2[3] = 0;
|
|
w3[0] = 0;
|
|
w3[1] = 0;
|
|
w3[2] = 0;
|
|
w3[3] = 0;
|
|
|
|
sha1_hmac_update_64 (&sha1_hmac_ctx2, w0, w1, w2, w3, 4);
|
|
|
|
sha1_hmac_final (&sha1_hmac_ctx2);
|
|
|
|
tmps[gid].dgst[i + 0] = sha1_hmac_ctx2.opad.h[0];
|
|
tmps[gid].dgst[i + 1] = sha1_hmac_ctx2.opad.h[1];
|
|
tmps[gid].dgst[i + 2] = sha1_hmac_ctx2.opad.h[2];
|
|
tmps[gid].dgst[i + 3] = sha1_hmac_ctx2.opad.h[3];
|
|
tmps[gid].dgst[i + 4] = sha1_hmac_ctx2.opad.h[4];
|
|
|
|
tmps[gid].out[i + 0] = tmps[gid].dgst[i + 0];
|
|
tmps[gid].out[i + 1] = tmps[gid].dgst[i + 1];
|
|
tmps[gid].out[i + 2] = tmps[gid].dgst[i + 2];
|
|
tmps[gid].out[i + 3] = tmps[gid].dgst[i + 3];
|
|
tmps[gid].out[i + 4] = tmps[gid].dgst[i + 4];
|
|
}
|
|
}
|
|
|
|
__kernel void m12000_loop (KERN_ATTR_TMPS_ESALT (pbkdf2_sha1_tmp_t, pbkdf2_sha1_t))
|
|
{
|
|
const u64 gid = get_global_id (0);
|
|
|
|
if ((gid * VECT_SIZE) >= gid_max) return;
|
|
|
|
u32x ipad[5];
|
|
u32x opad[5];
|
|
|
|
ipad[0] = packv (tmps, ipad, gid, 0);
|
|
ipad[1] = packv (tmps, ipad, gid, 1);
|
|
ipad[2] = packv (tmps, ipad, gid, 2);
|
|
ipad[3] = packv (tmps, ipad, gid, 3);
|
|
ipad[4] = packv (tmps, ipad, gid, 4);
|
|
|
|
opad[0] = packv (tmps, opad, gid, 0);
|
|
opad[1] = packv (tmps, opad, gid, 1);
|
|
opad[2] = packv (tmps, opad, gid, 2);
|
|
opad[3] = packv (tmps, opad, gid, 3);
|
|
opad[4] = packv (tmps, opad, gid, 4);
|
|
|
|
for (u32 i = 0; i < 4; i += 5)
|
|
{
|
|
u32x dgst[5];
|
|
u32x out[5];
|
|
|
|
dgst[0] = packv (tmps, dgst, gid, i + 0);
|
|
dgst[1] = packv (tmps, dgst, gid, i + 1);
|
|
dgst[2] = packv (tmps, dgst, gid, i + 2);
|
|
dgst[3] = packv (tmps, dgst, gid, i + 3);
|
|
dgst[4] = packv (tmps, dgst, gid, i + 4);
|
|
|
|
out[0] = packv (tmps, out, gid, i + 0);
|
|
out[1] = packv (tmps, out, gid, i + 1);
|
|
out[2] = packv (tmps, out, gid, i + 2);
|
|
out[3] = packv (tmps, out, gid, i + 3);
|
|
out[4] = packv (tmps, out, gid, i + 4);
|
|
|
|
for (u32 j = 0; j < loop_cnt; j++)
|
|
{
|
|
u32x w0[4];
|
|
u32x w1[4];
|
|
u32x w2[4];
|
|
u32x w3[4];
|
|
|
|
w0[0] = dgst[0];
|
|
w0[1] = dgst[1];
|
|
w0[2] = dgst[2];
|
|
w0[3] = dgst[3];
|
|
w1[0] = dgst[4];
|
|
w1[1] = 0x80000000;
|
|
w1[2] = 0;
|
|
w1[3] = 0;
|
|
w2[0] = 0;
|
|
w2[1] = 0;
|
|
w2[2] = 0;
|
|
w2[3] = 0;
|
|
w3[0] = 0;
|
|
w3[1] = 0;
|
|
w3[2] = 0;
|
|
w3[3] = (64 + 20) * 8;
|
|
|
|
hmac_sha1_run_V (w0, w1, w2, w3, ipad, opad, dgst);
|
|
|
|
out[0] ^= dgst[0];
|
|
out[1] ^= dgst[1];
|
|
out[2] ^= dgst[2];
|
|
out[3] ^= dgst[3];
|
|
out[4] ^= dgst[4];
|
|
}
|
|
|
|
unpackv (tmps, dgst, gid, i + 0, dgst[0]);
|
|
unpackv (tmps, dgst, gid, i + 1, dgst[1]);
|
|
unpackv (tmps, dgst, gid, i + 2, dgst[2]);
|
|
unpackv (tmps, dgst, gid, i + 3, dgst[3]);
|
|
unpackv (tmps, dgst, gid, i + 4, dgst[4]);
|
|
|
|
unpackv (tmps, out, gid, i + 0, out[0]);
|
|
unpackv (tmps, out, gid, i + 1, out[1]);
|
|
unpackv (tmps, out, gid, i + 2, out[2]);
|
|
unpackv (tmps, out, gid, i + 3, out[3]);
|
|
unpackv (tmps, out, gid, i + 4, out[4]);
|
|
}
|
|
}
|
|
|
|
__kernel void m12000_comp (KERN_ATTR_TMPS_ESALT (pbkdf2_sha1_tmp_t, pbkdf2_sha1_t))
|
|
{
|
|
/**
|
|
* base
|
|
*/
|
|
|
|
const u64 gid = get_global_id (0);
|
|
|
|
if (gid >= gid_max) return;
|
|
|
|
const u64 lid = get_local_id (0);
|
|
|
|
const u32 r0 = tmps[gid].out[DGST_R0];
|
|
const u32 r1 = tmps[gid].out[DGST_R1];
|
|
const u32 r2 = tmps[gid].out[DGST_R2];
|
|
const u32 r3 = tmps[gid].out[DGST_R3];
|
|
|
|
#define il_pos 0
|
|
|
|
#include COMPARE_M
|
|
}
|