mirror of
https://github.com/etesync/android
synced 2025-03-06 18:27:05 +00:00
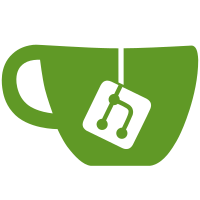
It seems like this is the only (semi) sane way to get base64 to work on both Android and local tests.
31 lines
1.2 KiB
Java
31 lines
1.2 KiB
Java
package com.etesync.syncadapter;
|
|
|
|
import com.etesync.syncadapter.utils.Base64;
|
|
import com.google.gson.Gson;
|
|
import com.google.gson.GsonBuilder;
|
|
import com.google.gson.JsonDeserializationContext;
|
|
import com.google.gson.JsonDeserializer;
|
|
import com.google.gson.JsonElement;
|
|
import com.google.gson.JsonParseException;
|
|
import com.google.gson.JsonPrimitive;
|
|
import com.google.gson.JsonSerializationContext;
|
|
import com.google.gson.JsonSerializer;
|
|
|
|
import java.lang.reflect.Type;
|
|
|
|
public class GsonHelper {
|
|
public static final Gson gson = new GsonBuilder().registerTypeHierarchyAdapter(byte[].class,
|
|
new ByteArrayToBase64TypeAdapter()).create();
|
|
|
|
// Using Android's base64 libraries. This can be replaced with any base64 library.
|
|
private static class ByteArrayToBase64TypeAdapter implements JsonSerializer<byte[]>, JsonDeserializer<byte[]> {
|
|
public byte[] deserialize(JsonElement json, Type typeOfT, JsonDeserializationContext context) throws JsonParseException {
|
|
return Base64.decode(json.getAsString(), Base64.NO_WRAP);
|
|
}
|
|
|
|
public JsonElement serialize(byte[] src, Type typeOfSrc, JsonSerializationContext context) {
|
|
return new JsonPrimitive(Base64.encodeToString(src, Base64.NO_WRAP));
|
|
}
|
|
}
|
|
}
|