mirror of
https://github.com/etesync/android
synced 2024-11-15 20:38:58 +00:00
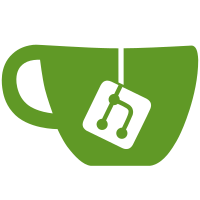
This is a monster commit because to be honest, it's a monster change. It was impossible to do it in smaller steps because things just wouldn't compile. We couldn't do the migration step by step because they moved to Kotlin which was causing a lot of troubles. Now we are all on Kotlin, so things should hopefully work just fine.
171 lines
6.2 KiB
Groovy
171 lines
6.2 KiB
Groovy
/*
|
|
* Copyright (c) Ricki Hirner (bitfire web engineering).
|
|
* All rights reserved. This program and the accompanying materials
|
|
* are made available under the terms of the GNU Public License v3.0
|
|
* which accompanies this distribution, and is available at
|
|
* http://www.gnu.org/licenses/gpl.html
|
|
*/
|
|
|
|
apply plugin: 'com.android.application'
|
|
apply plugin: 'kotlin-android-extensions'
|
|
apply plugin: 'kotlin-android'
|
|
apply plugin: 'kotlin-kapt'
|
|
|
|
android {
|
|
compileSdkVersion 28
|
|
buildToolsVersion '28.0.3'
|
|
|
|
defaultConfig {
|
|
applicationId "com.etesync.syncadapter"
|
|
|
|
minSdkVersion 19
|
|
targetSdkVersion 26
|
|
|
|
versionCode 43
|
|
versionName "0.22.6"
|
|
|
|
buildConfigField "long", "buildTime", System.currentTimeMillis() + "L"
|
|
buildConfigField "boolean", "customCerts", "true"
|
|
}
|
|
|
|
buildTypes {
|
|
debug {
|
|
minifyEnabled true
|
|
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.txt'
|
|
|
|
/*
|
|
* To override the server's url (for example when developing),
|
|
* create file gradle.properties in ~/.gradle/ with this content:
|
|
*
|
|
* appDebugRemoteUrl="http://localserver:8080/"
|
|
*/
|
|
if (project.hasProperty('appDebugRemoteUrl')) {
|
|
buildConfigField 'String', 'DEBUG_REMOTE_URL', appDebugRemoteUrl
|
|
} else {
|
|
buildConfigField 'String', 'DEBUG_REMOTE_URL', 'null'
|
|
}
|
|
}
|
|
release {
|
|
minifyEnabled true
|
|
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.txt'
|
|
buildConfigField 'String', 'DEBUG_REMOTE_URL', 'null'
|
|
|
|
// Configure Kotlin compiler optimisations for releases
|
|
kotlinOptions {
|
|
freeCompilerArgs = [
|
|
'-Xno-param-assertions',
|
|
'-Xno-call-assertions',
|
|
'-Xno-receiver-assertions'
|
|
]
|
|
}
|
|
}
|
|
}
|
|
|
|
lintOptions {
|
|
disable 'GoogleAppIndexingWarning' // we don't need Google indexing, thanks
|
|
disable 'GradleDependency'
|
|
disable 'GradleDynamicVersion'
|
|
disable 'IconColors'
|
|
disable 'IconLauncherShape'
|
|
disable 'IconMissingDensityFolder'
|
|
disable 'ImpliedQuantity', 'MissingQuantity'
|
|
disable 'MissingTranslation', 'ExtraTranslation' // translations from Transifex are not always up to date
|
|
disable 'Recycle' // doesn't understand Lombok's @Cleanup
|
|
disable 'RtlEnabled'
|
|
disable 'RtlHardcoded'
|
|
disable 'Typos'
|
|
disable "RestrictedApi" // https://code.google.com/p/android/issues/detail?id=230387
|
|
}
|
|
|
|
dexOptions {
|
|
preDexLibraries = true
|
|
// dexInProcess requires much RAM, which is not available on all dev systems
|
|
dexInProcess = false
|
|
javaMaxHeapSize "2g"
|
|
}
|
|
|
|
packagingOptions {
|
|
exclude 'LICENSE'
|
|
exclude 'META-INF/LICENSE.txt'
|
|
exclude 'META-INF/NOTICE.txt'
|
|
}
|
|
|
|
defaultConfig {
|
|
testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner"
|
|
}
|
|
|
|
/*
|
|
* To sign release build, create file gradle.properties in ~/.gradle/ with this content:
|
|
*
|
|
* signingStoreLocation=/home/key.store
|
|
* signingKeyAlias=alias
|
|
*
|
|
* and set the KSTOREPWD env var to the store and key passwords (should be the same)
|
|
*/
|
|
if (project.hasProperty('signingStoreLocation') &&
|
|
project.hasProperty('signingKeyAlias')) {
|
|
println "Found sign properties in gradle.properties! Signing build…"
|
|
|
|
signingConfigs {
|
|
release {
|
|
storeFile file(signingStoreLocation)
|
|
storePassword System.getenv("KSTOREPWD")
|
|
keyAlias signingKeyAlias
|
|
keyPassword System.getenv("KSTOREPWD")
|
|
|
|
}
|
|
}
|
|
buildTypes.release.signingConfig = signingConfigs.release
|
|
} else {
|
|
buildTypes.release.signingConfig = null
|
|
}
|
|
compileOptions {
|
|
sourceCompatibility JavaVersion.VERSION_1_8
|
|
targetCompatibility JavaVersion.VERSION_1_8
|
|
}
|
|
}
|
|
|
|
dependencies {
|
|
def acraVersion = '5.2.1'
|
|
implementation "ch.acra:acra-mail:$acraVersion"
|
|
implementation "ch.acra:acra-toast:$acraVersion"
|
|
def supportVersion = '27.1.1'
|
|
implementation "com.android.support:support-core-ui:$supportVersion"
|
|
implementation "com.android.support:support-compat:$supportVersion"
|
|
implementation "com.android.support:support-fragment:$supportVersion"
|
|
implementation "com.android.support:appcompat-v7:$supportVersion"
|
|
implementation "com.android.support:cardview-v7:$supportVersion"
|
|
implementation "com.android.support:design:$supportVersion"
|
|
implementation "com.android.support:preference-v14:$supportVersion"
|
|
compile 'com.github.yukuku:ambilwarna:2.0.1'
|
|
compile('com.github.worker8:tourguide:1.0.17-SNAPSHOT@aar') {
|
|
transitive = true
|
|
}
|
|
compile 'io.requery:requery:1.5.0'
|
|
compile 'io.requery:requery-android:1.5.0'
|
|
compile 'io.requery:requery-kotlin:1.5.0'
|
|
kapt 'io.requery:requery-processor:1.5.0'
|
|
compile 'com.madgag.spongycastle:core:1.54.0.0'
|
|
compile 'com.madgag.spongycastle:prov:1.54.0.0'
|
|
compile 'com.google.code.gson:gson:1.7.2'
|
|
compile 'com.squareup.okhttp3:logging-interceptor:3.8.0'
|
|
compile 'org.apache.commons:commons-collections4:4.1'
|
|
compileOnly 'org.projectlombok:lombok:1.16.20'
|
|
annotationProcessor 'org.projectlombok:lombok:1.16.20'
|
|
compile 'net.cachapa.expandablelayout:expandablelayout:2.9.2'
|
|
compile project(':cert4android')
|
|
compile project(':ical4android')
|
|
compile project(':vcard4android')
|
|
// for tests
|
|
androidTestCompile('com.android.support.test:runner:0.5') {
|
|
exclude group: 'com.android.support', module: 'support-annotations'
|
|
}
|
|
androidTestCompile('com.android.support.test:rules:0.5') {
|
|
exclude group: 'com.android.support', module: 'support-annotations'
|
|
}
|
|
androidTestCompile 'junit:junit:4.12'
|
|
androidTestCompile 'com.squareup.okhttp3:mockwebserver:3.8.0'
|
|
testCompile 'junit:junit:4.12'
|
|
testCompile 'com.squareup.okhttp3:mockwebserver:3.8.0'
|
|
}
|