mirror of
https://github.com/etesync/android
synced 2024-11-16 04:49:06 +00:00
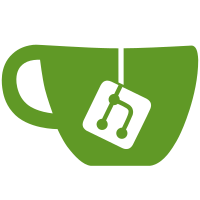
This will make it easier to identify and fix crashes. Until now we relied on user to automatically figure out if the app has crashed and gather debug info manually. This didn't work well, especially in places like "import" where they just assumed the import finished successfully if there was a crash. This change makes it so whenever there's a crash, the email app is opened with a template email and the stack trace attached. This should make it easier for us to detect and fix issues. Important to note: nothing is sent automatically.
158 lines
5.4 KiB
Groovy
158 lines
5.4 KiB
Groovy
/*
|
|
* Copyright (c) Ricki Hirner (bitfire web engineering).
|
|
* All rights reserved. This program and the accompanying materials
|
|
* are made available under the terms of the GNU Public License v3.0
|
|
* which accompanies this distribution, and is available at
|
|
* http://www.gnu.org/licenses/gpl.html
|
|
*/
|
|
|
|
apply plugin: 'com.android.application'
|
|
|
|
android {
|
|
compileSdkVersion 27
|
|
buildToolsVersion '27.0.1'
|
|
|
|
defaultConfig {
|
|
applicationId "com.etesync.syncadapter"
|
|
|
|
minSdkVersion 16
|
|
targetSdkVersion 25
|
|
|
|
versionCode 24
|
|
versionName "0.19.6"
|
|
|
|
buildConfigField "long", "buildTime", System.currentTimeMillis() + "L"
|
|
buildConfigField "boolean", "customCerts", "true"
|
|
}
|
|
|
|
buildTypes {
|
|
debug {
|
|
minifyEnabled true
|
|
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.txt'
|
|
|
|
/*
|
|
* To override the server's url (for example when developing),
|
|
* create file gradle.properties in ~/.gradle/ with this content:
|
|
*
|
|
* appDebugRemoteUrl="http://localserver:8080/"
|
|
*/
|
|
if (project.hasProperty('appDebugRemoteUrl')) {
|
|
buildConfigField 'String', 'DEBUG_REMOTE_URL', appDebugRemoteUrl
|
|
} else {
|
|
buildConfigField 'String', 'DEBUG_REMOTE_URL', 'null'
|
|
}
|
|
}
|
|
release {
|
|
minifyEnabled true
|
|
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.txt'
|
|
buildConfigField 'String', 'DEBUG_REMOTE_URL', 'null'
|
|
}
|
|
}
|
|
|
|
lintOptions {
|
|
disable 'GoogleAppIndexingWarning' // we don't need Google indexing, thanks
|
|
disable 'GradleDependency'
|
|
disable 'GradleDynamicVersion'
|
|
disable 'IconColors'
|
|
disable 'IconLauncherShape'
|
|
disable 'IconMissingDensityFolder'
|
|
disable 'ImpliedQuantity', 'MissingQuantity'
|
|
disable 'MissingTranslation', 'ExtraTranslation' // translations from Transifex are not always up to date
|
|
disable 'Recycle' // doesn't understand Lombok's @Cleanup
|
|
disable 'RtlEnabled'
|
|
disable 'RtlHardcoded'
|
|
disable 'Typos'
|
|
disable "RestrictedApi" // https://code.google.com/p/android/issues/detail?id=230387
|
|
}
|
|
|
|
dexOptions {
|
|
preDexLibraries = true
|
|
// dexInProcess requires much RAM, which is not available on all dev systems
|
|
dexInProcess = false
|
|
javaMaxHeapSize "2g"
|
|
}
|
|
|
|
packagingOptions {
|
|
exclude 'LICENSE'
|
|
exclude 'META-INF/LICENSE.txt'
|
|
exclude 'META-INF/NOTICE.txt'
|
|
}
|
|
|
|
defaultConfig {
|
|
testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner"
|
|
}
|
|
|
|
/*
|
|
* To sign release build, create file gradle.properties in ~/.gradle/ with this content:
|
|
*
|
|
* signingStoreLocation=/home/key.store
|
|
* signingKeyAlias=alias
|
|
*
|
|
* and set the KSTOREPWD env var to the store and key passwords (should be the same)
|
|
*/
|
|
if (project.hasProperty('signingStoreLocation') &&
|
|
project.hasProperty('signingKeyAlias')) {
|
|
println "Found sign properties in gradle.properties! Signing build…"
|
|
|
|
signingConfigs {
|
|
release {
|
|
storeFile file(signingStoreLocation)
|
|
storePassword System.getenv("KSTOREPWD")
|
|
keyAlias signingKeyAlias
|
|
keyPassword System.getenv("KSTOREPWD")
|
|
|
|
}
|
|
}
|
|
buildTypes.release.signingConfig = signingConfigs.release
|
|
} else {
|
|
buildTypes.release.signingConfig = null
|
|
}
|
|
|
|
}
|
|
|
|
dependencies {
|
|
def acraVersion = '5.0.1'
|
|
implementation "ch.acra:acra-mail:$acraVersion"
|
|
implementation "ch.acra:acra-toast:$acraVersion"
|
|
|
|
compile project(':cert4android')
|
|
compile project(':ical4android')
|
|
compile project(':vcard4android')
|
|
|
|
compile 'com.android.support:appcompat-v7:26.+'
|
|
compile 'com.android.support:cardview-v7:26.+'
|
|
compile 'com.android.support:design:26.+'
|
|
compile 'com.android.support:preference-v14:26.+'
|
|
|
|
compile 'com.github.yukuku:ambilwarna:2.0.1'
|
|
compile ('com.github.worker8:tourguide:1.0.17-SNAPSHOT@aar'){
|
|
transitive=true
|
|
}
|
|
|
|
compile 'io.requery:requery:1.3.1'
|
|
compile 'io.requery:requery-android:1.3.1'
|
|
annotationProcessor 'io.requery:requery-processor:1.3.1'
|
|
|
|
compile group: 'com.madgag.spongycastle', name: 'core', version: '1.54.0.0'
|
|
compile group: 'com.madgag.spongycastle', name: 'prov', version: '1.54.0.0'
|
|
compile group: 'com.google.code.gson', name: 'gson', version: '1.7.2'
|
|
compile 'com.squareup.okhttp3:logging-interceptor:3.8.0'
|
|
compile 'org.apache.commons:commons-collections4:4.1'
|
|
compileOnly 'org.projectlombok:lombok:1.16.20'
|
|
annotationProcessor 'org.projectlombok:lombok:1.16.20'
|
|
compile 'net.cachapa.expandablelayout:expandablelayout:2.9.2'
|
|
|
|
// for tests
|
|
androidTestCompile('com.android.support.test:runner:0.5') {
|
|
exclude group: 'com.android.support', module: 'support-annotations'
|
|
}
|
|
androidTestCompile('com.android.support.test:rules:0.5') {
|
|
exclude group: 'com.android.support', module: 'support-annotations'
|
|
}
|
|
androidTestCompile 'junit:junit:4.12'
|
|
androidTestCompile 'com.squareup.okhttp3:mockwebserver:3.8.0'
|
|
|
|
testCompile 'junit:junit:4.12'
|
|
testCompile 'com.squareup.okhttp3:mockwebserver:3.8.0'
|
|
}
|