mirror of
https://github.com/bitcoinbook/bitcoinbook
synced 2024-11-15 20:49:21 +00:00
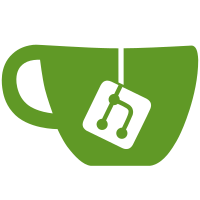
The commit ab5ae32bae
is the last commit
for the second edition, so all changes since then are dropped except for
several commits for the third edition authored by Andreas Antonopoulos.
No attempt is made to remove CC-BY-SA or other licensed content present
in the already-published first or second editions.
This revert may itself be reverted for versions of the book published
under CC-BY-SA.
47 lines
2.0 KiB
Python
47 lines
2.0 KiB
Python
from __future__ import print_function
|
|
import bitcoin
|
|
|
|
# Generate a random private key
|
|
valid_private_key = False
|
|
while not valid_private_key:
|
|
private_key = bitcoin.random_key()
|
|
decoded_private_key = bitcoin.decode_privkey(private_key, 'hex')
|
|
valid_private_key = 0 < decoded_private_key < bitcoin.N
|
|
|
|
print("Private Key (hex) is: ", private_key)
|
|
print("Private Key (decimal) is: ", decoded_private_key)
|
|
|
|
# Convert private key to WIF format
|
|
wif_encoded_private_key = bitcoin.encode_privkey(decoded_private_key, 'wif')
|
|
print("Private Key (WIF) is: ", wif_encoded_private_key)
|
|
|
|
# Add suffix "01" to indicate a compressed private key
|
|
compressed_private_key = private_key + '01'
|
|
print("Private Key Compressed (hex) is: ", compressed_private_key)
|
|
|
|
# Generate a WIF format from the compressed private key (WIF-compressed)
|
|
wif_compressed_private_key = bitcoin.encode_privkey(
|
|
bitcoin.decode_privkey(compressed_private_key, 'hex'), 'wif_compressed')
|
|
print("Private Key (WIF-Compressed) is: ", wif_compressed_private_key)
|
|
|
|
# Multiply the EC generator point G with the private key to get a public key point
|
|
public_key = bitcoin.fast_multiply(bitcoin.G, decoded_private_key)
|
|
print("Public Key (x,y) coordinates is:", public_key)
|
|
|
|
# Encode as hex, prefix 04
|
|
hex_encoded_public_key = bitcoin.encode_pubkey(public_key, 'hex')
|
|
print("Public Key (hex) is:", hex_encoded_public_key)
|
|
|
|
# Compress public key, adjust prefix depending on whether y is even or odd
|
|
(public_key_x, public_key_y) = public_key
|
|
compressed_prefix = '02' if (public_key_y % 2) == 0 else '03'
|
|
hex_compressed_public_key = compressed_prefix + (bitcoin.encode(public_key_x, 16).zfill(64))
|
|
print("Compressed Public Key (hex) is:", hex_compressed_public_key)
|
|
|
|
# Generate Bitcoin address from public key
|
|
print("Bitcoin Address (b58check) is:", bitcoin.pubkey_to_address(public_key))
|
|
|
|
# Generate compressed Bitcoin address from compressed public key
|
|
print("Compressed Bitcoin Address (b58check) is:",
|
|
bitcoin.pubkey_to_address(hex_compressed_public_key))
|