mirror of
https://github.com/bitcoinbook/bitcoinbook
synced 2024-11-15 20:49:21 +00:00
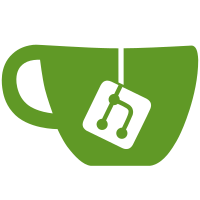
The commit ab5ae32bae
is the last commit
for the second edition, so all changes since then are dropped except for
several commits for the third edition authored by Andreas Antonopoulos.
No attempt is made to remove CC-BY-SA or other licensed content present
in the already-published first or second editions.
This revert may itself be reverted for versions of the book published
under CC-BY-SA.
60 lines
1.8 KiB
Python
60 lines
1.8 KiB
Python
import ecdsa
|
|
import os
|
|
|
|
# secp256k1, http://www.oid-info.com/get/1.3.132.0.10
|
|
_p = 0xFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFEFFFFFC2F
|
|
_r = 0xFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFEBAAEDCE6AF48A03BBFD25E8CD0364141
|
|
_b = 0x0000000000000000000000000000000000000000000000000000000000000007
|
|
_a = 0x0000000000000000000000000000000000000000000000000000000000000000
|
|
_Gx = 0x79BE667EF9DCBBAC55A06295CE870B07029BFCDB2DCE28D959F2815B16F81798
|
|
_Gy = 0x483ada7726a3c4655da4fbfc0e1108a8fd17b448a68554199c47d08ffb10d4b8
|
|
curve_secp256k1 = ecdsa.ellipticcurve.CurveFp(_p, _a, _b)
|
|
generator_secp256k1 = ecdsa.ellipticcurve.Point(curve_secp256k1, _Gx, _Gy, _r)
|
|
oid_secp256k1 = (1, 3, 132, 0, 10)
|
|
SECP256k1 = ecdsa.curves.Curve("SECP256k1", curve_secp256k1,
|
|
generator_secp256k1, oid_secp256k1)
|
|
ec_order = _r
|
|
|
|
curve = curve_secp256k1
|
|
generator = generator_secp256k1
|
|
|
|
|
|
def random_secret():
|
|
convert_to_int = lambda array: int("".join(array).encode("hex"), 16)
|
|
|
|
# Collect 256 bits of random data from the OS's cryptographically secure
|
|
# random number generator
|
|
byte_array = os.urandom(32)
|
|
|
|
return convert_to_int(byte_array)
|
|
|
|
|
|
def get_point_pubkey(point):
|
|
if (point.y() % 2) == 1:
|
|
key = '03' + '%064x' % point.x()
|
|
else:
|
|
key = '02' + '%064x' % point.x()
|
|
return key.decode('hex')
|
|
|
|
|
|
def get_point_pubkey_uncompressed(point):
|
|
key = ('04' +
|
|
'%064x' % point.x() +
|
|
'%064x' % point.y())
|
|
return key.decode('hex')
|
|
|
|
|
|
# Generate a new private key.
|
|
secret = random_secret()
|
|
print("Secret: ", secret)
|
|
|
|
# Get the public key point.
|
|
point = secret * generator
|
|
print("EC point:", point)
|
|
|
|
print("BTC public key:", get_point_pubkey(point).encode("hex"))
|
|
|
|
# Given the point (x, y) we can create the object using:
|
|
point1 = ecdsa.ellipticcurve.Point(curve, point.x(), point.y(), ec_order)
|
|
assert(point1 == point)
|