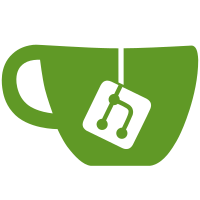
1. Remove appmenus - regenerate them at installation time (start the template for that) 2. Remove volatile.img - regenerate it at installation time This way, the only real data carried in template rpm is root.img.
114 lines
3.1 KiB
Bash
Executable File
114 lines
3.1 KiB
Bash
Executable File
#!/bin/sh
|
|
|
|
# ------------------------------------------------------------------------------
|
|
# Configurations and Conditionals
|
|
# ------------------------------------------------------------------------------
|
|
export CLEANIMG="$1"
|
|
export NAME="$2"
|
|
export LC_ALL=POSIX
|
|
|
|
. ./builder_setup >/dev/null
|
|
. ./umount_kill.sh >/dev/null
|
|
|
|
if [ "$VERBOSE" -ge 2 -o "$DEBUG" == "1" ]; then
|
|
set -x
|
|
else
|
|
set -e
|
|
fi
|
|
|
|
if [ $# -eq 0 ]; then
|
|
echo "usage $0 <clean_image_file> <template_name>"
|
|
exit
|
|
fi
|
|
|
|
if [ "x$CLEANIMG" = x ]; then
|
|
echo "Image file not specified!"
|
|
exit 1
|
|
fi
|
|
|
|
if [ "x$NAME" = x ]; then
|
|
echo "Name not given!"
|
|
exit 1
|
|
fi
|
|
|
|
ID=$(id -ur)
|
|
|
|
if [ $ID != 0 ] ; then
|
|
echo "This script should be run as root user."
|
|
exit 1
|
|
fi
|
|
|
|
if [ "$VERBOSE" == "1" ]; then
|
|
export YUM_OPTS="$YUM_OPTS -q"
|
|
fi
|
|
|
|
# ------------------------------------------------------------------------------
|
|
# Cleanup function
|
|
# ------------------------------------------------------------------------------
|
|
function cleanup() {
|
|
errval=$?
|
|
trap - ERR
|
|
trap
|
|
umount_kill "$PWD/mnt" || true
|
|
exit $errval
|
|
}
|
|
trap cleanup ERR
|
|
|
|
# ------------------------------------------------------------------------------
|
|
# Mount qubeized_image
|
|
# ------------------------------------------------------------------------------
|
|
export IMG="qubeized_images/$NAME-root.img"
|
|
|
|
echo "--> Copying $CLEANIMG to $IMG..."
|
|
cp "$CLEANIMG" "$IMG" || exit 1
|
|
|
|
echo "--> Mounting $IMG"
|
|
mkdir -p mnt
|
|
mount -o loop "$IMG" mnt || exit 1
|
|
export INSTALLDIR=mnt
|
|
|
|
# ------------------------------------------------------------------------------
|
|
# Run qubeize script
|
|
# ------------------------------------------------------------------------------
|
|
"$SCRIPTSDIR/04_install_qubes.sh"
|
|
|
|
# ------------------------------------------------------------------------------
|
|
# Create App Menus
|
|
# ------------------------------------------------------------------------------
|
|
echo "--> Choosing appmenus whitelists..."
|
|
rm -f appmenus
|
|
if [ -d "appmenus_${DIST}_${TEMPLATE_FLAVOR}" ]; then
|
|
ln -s "appmenus_${DIST}_${TEMPLATE_FLAVOR}" appmenus
|
|
elif [ -d "appmenus_$DIST" ]; then
|
|
ln -s "appmenus_$DIST" appmenus
|
|
else
|
|
ln -s "appmenus_generic" appmenus
|
|
fi
|
|
|
|
# ------------------------------------------------------------------------------
|
|
# Link directories so they can be mounted
|
|
# ------------------------------------------------------------------------------
|
|
echo "--> Linking /home to /rw/home..."
|
|
mv mnt/home mnt/home.orig
|
|
ln -sf /rw/home mnt/home
|
|
|
|
echo "--> Linking /usr/local to /rw/usrlocal..."
|
|
mv mnt/usr/local mnt/usr/local.orig
|
|
ln -sf /rw/usrlocal mnt/usr/local
|
|
|
|
if [ -e mnt/etc/sysconfig/i18n ]; then
|
|
echo "--> Setting up default locale..."
|
|
echo LC_CTYPE=en_US.UTF-8 > mnt/etc/sysconfig/i18n
|
|
fi
|
|
|
|
# ------------------------------------------------------------------------------
|
|
# Finsh - unmount image
|
|
# ------------------------------------------------------------------------------
|
|
echo "--> Unmounting $IMG"
|
|
umount_kill "$PWD/mnt" || true
|
|
|
|
echo "Qubeized image stored at: $IMG"
|
|
|
|
echo "Reducing image size (calling cleanup_image)..."
|
|
./cleanup_image "$IMG"
|