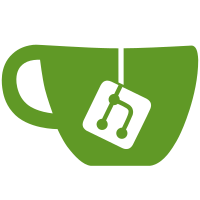
This commit avoids the issue where the UEFI boot uses the Fedora 23 kernel as opposed to the Qubes OS kernel.
118 lines
4.4 KiB
Cheetah
118 lines
4.4 KiB
Cheetah
<%page args="kernels, runtime_img, basearch, outroot, product, isolabel"/>
|
|
<%
|
|
configdir="tmp/config_files/x86"
|
|
SYSLINUXDIR="usr/share/syslinux"
|
|
PXEBOOTDIR="images/pxeboot"
|
|
BOOTDIR="isolinux"
|
|
KERNELDIR=PXEBOOTDIR
|
|
LIVEDIR="LiveOS"
|
|
EXTRAKERNELS="extrakernels"
|
|
from distutils.version import LooseVersion
|
|
|
|
## Don't allow spaces or escape characters in the iso label
|
|
def valid_label(ch):
|
|
return ch.isalnum() or ch == '_'
|
|
|
|
isolabel = ''.join(ch if valid_label(ch) else '-' for ch in isolabel)
|
|
%>
|
|
|
|
mkdir ${LIVEDIR}
|
|
install ${runtime_img} ${LIVEDIR}/squashfs.img
|
|
treeinfo stage2 mainimage ${LIVEDIR}/squashfs.img
|
|
|
|
## install bootloader and config files
|
|
mkdir ${BOOTDIR}
|
|
install ${SYSLINUXDIR}/isolinux.bin ${BOOTDIR}
|
|
install ${SYSLINUXDIR}/vesamenu.c32 ${BOOTDIR}
|
|
install ${SYSLINUXDIR}/mboot.c32 ${BOOTDIR}
|
|
install ${SYSLINUXDIR}/ldlinux.c32 ${BOOTDIR}
|
|
install ${SYSLINUXDIR}/libcom32.c32 ${BOOTDIR}
|
|
install ${SYSLINUXDIR}/libutil.c32 ${BOOTDIR}
|
|
install boot/xen*gz ${BOOTDIR}/xen.gz
|
|
install ${configdir}/isolinux.cfg ${BOOTDIR}
|
|
install ${configdir}/boot.msg ${BOOTDIR}
|
|
install ${configdir}/grub.conf ${BOOTDIR}
|
|
install usr/share/anaconda/boot/syslinux-splash.png ${BOOTDIR}/splash.png
|
|
install boot/memtest* ${BOOTDIR}/memtest
|
|
|
|
## install kernels
|
|
mkdir ${KERNELDIR}
|
|
<%
|
|
sortedkernels = sorted(kernels, key=lambda k: LooseVersion(k['version']))
|
|
sortedkernels = [ kernel for kernel in sortedkernels if 'qubes' in kernel.version ]
|
|
%>
|
|
|
|
%for kernel in sortedkernels:
|
|
# Use short kernel version because of ISO9660 filename length limitation
|
|
<%
|
|
shortkver = kernel.version.replace('.pvops.qubes','').replace('.x86_64','')
|
|
%>
|
|
%if kernel.flavor:
|
|
## i386 PAE
|
|
installkernel images-xen ${kernel.path} ${KERNELDIR}/vmlinuz-${kernel.flavor}
|
|
installinitrd images-xen ${kernel.initrd.path} ${KERNELDIR}/initrd-${kernel.flavor}.img
|
|
%if doupgrade:
|
|
installupgradeinitrd images-xen ${kernel.upgrade.path} ${KERNELDIR}/upgrade-${kernel.flavor}.img
|
|
%endif
|
|
%else:
|
|
## normal i386, x86_64
|
|
installkernel images-${basearch} ${kernel.path} ${KERNELDIR}/vmlinuz
|
|
installinitrd images-${basearch} ${kernel.initrd.path} ${KERNELDIR}/initrd.img
|
|
%if doupgrade:
|
|
installupgradeinitrd images-${basearch} ${kernel.upgrade.path} ${KERNELDIR}/upgrade.img
|
|
%endif
|
|
%endif
|
|
installkernel images-alt-${shortkver} ${kernel.path} ${BOOTDIR}/vmlinuz-${shortkver}
|
|
installinitrd images-alt-${shortkver} ${kernel.initrd.path} ${BOOTDIR}/initrd-${shortkver}.img
|
|
|
|
<% latestkver = kernel.version %>
|
|
|
|
%endfor
|
|
|
|
## configure bootloader
|
|
replace @VERSION@ ${product.version} ${BOOTDIR}/grub.conf ${BOOTDIR}/isolinux.cfg ${BOOTDIR}/*.msg
|
|
replace @PRODUCT@ '${product.name}' ${BOOTDIR}/grub.conf ${BOOTDIR}/isolinux.cfg ${BOOTDIR}/*.msg
|
|
replace @ROOT@ 'inst.stage2=hd:LABEL=${isolabel|udev}' ${BOOTDIR}/isolinux.cfg
|
|
replace @EXTRAKERNELS@ '' ${BOOTDIR}/isolinux.cfg
|
|
|
|
hardlink ${KERNELDIR}/vmlinuz ${BOOTDIR}
|
|
hardlink ${KERNELDIR}/initrd.img ${BOOTDIR}
|
|
%if doupgrade:
|
|
hardlink ${KERNELDIR}/upgrade.img ${BOOTDIR}
|
|
%endif
|
|
%if basearch == 'x86_64':
|
|
treeinfo images-xen kernel ${KERNELDIR}/vmlinuz
|
|
treeinfo images-xen initrd ${KERNELDIR}/initrd.img
|
|
%if doupgrade:
|
|
treeinfo images-xen upgrade ${KERNELDIR}/upgrade.img
|
|
%endif
|
|
%endif
|
|
|
|
## WHeeeeeeee, EFI.
|
|
## We could remove the basearch restriction someday..
|
|
<% efiargs=""; efigraft=""; efihybrid="" %>
|
|
%if exists("boot/efi/EFI/*/gcdx64.efi") and basearch != 'i386':
|
|
<%
|
|
efiarch = 'X64' if basearch=='x86_64' else 'IA32'
|
|
efigraft="EFI/BOOT={0}/EFI/BOOT".format(outroot)
|
|
images = ["images/efiboot.img"]
|
|
for img in images:
|
|
efiargs += " -eltorito-alt-boot -e {0} -no-emul-boot".format(img)
|
|
efigraft += " {0}={1}/{0}".format(img,outroot)
|
|
efihybrid = "--uefi"
|
|
%>
|
|
<%include file="efi.tmpl" args="configdir=configdir, KERNELDIR=KERNELDIR, efiarch=efiarch, isolabel=isolabel, kver=latestkver"/>
|
|
%endif
|
|
|
|
## ## make boot.iso
|
|
## runcmd mkisofs -o ${outroot}/images/boot.iso \
|
|
## -b ${BOOTDIR}/isolinux.bin -c ${BOOTDIR}/boot.cat \
|
|
## -boot-load-size 4 -boot-info-table -no-emul-boot \
|
|
## ${efiargs} -R -J -V '${isolabel}' -T -graft-points \
|
|
## ${BOOTDIR}=${outroot}/${BOOTDIR} \
|
|
## ${KERNELDIR}=${outroot}/${KERNELDIR} \
|
|
## ${LIVEDIR}=${outroot}/${LIVEDIR} \
|
|
## ${efigraft}
|
|
## runcmd isohybrid ${efihybrid} ${outroot}/images/boot.iso
|
|
## treeinfo images-${basearch} boot.iso images/boot.iso
|